注意
单击此处下载完整的示例代码
知识蒸馏教程¶
创建于:2023 年 8 月 22 日 | 最后更新:2025 年 1 月 24 日 | 最后验证:2024 年 11 月 05 日
知识蒸馏是一种技术,可以将知识从大型、计算成本高的模型转移到较小的模型,而不会失去有效性。这允许在性能较低的硬件上部署,从而使评估更快、更有效。
在本教程中,我们将运行一系列实验,重点是通过使用更强大的网络作为教师来提高轻量级神经网络的准确性。轻量级网络的计算成本和速度将保持不变,我们的干预仅关注其权重,而不是其前向传播。这项技术的应用可以在无人机或手机等设备中找到。在本教程中,我们不使用任何外部软件包,因为我们所需的一切都可以在 torch
和 torchvision
中找到。
在本教程中,您将学习
如何修改模型类以提取隐藏表示并将其用于进一步计算
如何在 PyTorch 中修改常规训练循环,以包含额外的损失,例如,分类的交叉熵
如何通过使用更复杂的模型作为教师来提高轻量级模型的性能
先决条件¶
1 个 GPU,4GB 内存
PyTorch v2.0 或更高版本
CIFAR-10 数据集(由脚本下载并保存在名为
/data
的目录中)
import torch
import torch.nn as nn
import torch.optim as optim
import torchvision.transforms as transforms
import torchvision.datasets as datasets
# Check if the current `accelerator <https://pytorch.ac.cn/docs/stable/torch.html#accelerators>`__
# is available, and if not, use the CPU
device = torch.accelerator.current_accelerator().type if torch.accelerator.is_available() else "cpu"
print(f"Using {device} device")
Using cuda device
加载 CIFAR-10¶
CIFAR-10 是一个包含十个类别的流行图像数据集。我们的目标是为每个输入图像预测以下类别之一。
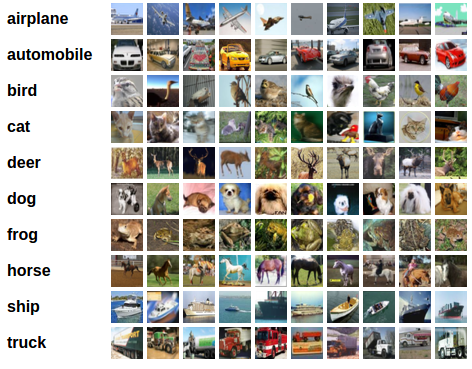
CIFAR-10 图像示例¶
输入图像是 RGB 格式,因此它们有 3 个通道,大小为 32x32 像素。基本上,每个图像由 3 x 32 x 32 = 3072 个介于 0 到 255 之间的数字描述。神经网络中的常见做法是对输入进行归一化,这样做有多种原因,包括避免常用激活函数中的饱和并提高数值稳定性。我们的归一化过程包括减去均值并除以每个通道的标准差。“mean=[0.485, 0.456, 0.406]” 和 “std=[0.229, 0.224, 0.225]” 张量已经计算出来,它们表示 CIFAR-10 预定义子集中每个通道的均值和标准差,该子集旨在作为训练集。请注意,我们如何在测试集中也使用这些值,而无需从头开始重新计算均值和标准差。这是因为网络是在通过减去和除以上述数字而产生的特征上训练的,我们希望保持一致性。此外,在现实生活中,我们将无法计算测试集的均值和标准差,因为根据我们的假设,这些数据在当时是不可访问的。
作为结尾,我们经常将此保留集称为验证集,并在优化模型在验证集上的性能后使用一个单独的集合(称为测试集)。这样做是为了避免基于单个指标的贪婪和有偏优化来选择模型。
# Below we are preprocessing data for CIFAR-10. We use an arbitrary batch size of 128.
transforms_cifar = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]),
])
# Loading the CIFAR-10 dataset:
train_dataset = datasets.CIFAR10(root='./data', train=True, download=True, transform=transforms_cifar)
test_dataset = datasets.CIFAR10(root='./data', train=False, download=True, transform=transforms_cifar)
0%| | 0.00/170M [00:00<?, ?B/s]
0%| | 754k/170M [00:00<00:22, 7.53MB/s]
4%|4 | 7.50M/170M [00:00<00:03, 42.8MB/s]
10%|9 | 17.0M/170M [00:00<00:02, 66.2MB/s]
16%|#5 | 26.7M/170M [00:00<00:01, 78.4MB/s]
22%|##1 | 36.7M/170M [00:00<00:01, 86.2MB/s]
27%|##7 | 46.8M/170M [00:00<00:01, 90.9MB/s]
33%|###3 | 56.7M/170M [00:00<00:01, 93.6MB/s]
39%|###8 | 66.5M/170M [00:00<00:01, 94.7MB/s]
45%|####4 | 76.2M/170M [00:00<00:00, 95.3MB/s]
51%|##### | 86.4M/170M [00:01<00:00, 97.2MB/s]
57%|#####7 | 97.4M/170M [00:01<00:00, 101MB/s]
64%|######3 | 108M/170M [00:01<00:00, 104MB/s]
70%|######9 | 119M/170M [00:01<00:00, 104MB/s]
76%|#######5 | 129M/170M [00:01<00:00, 96.3MB/s]
82%|########1 | 139M/170M [00:01<00:00, 92.1MB/s]
87%|########7 | 148M/170M [00:01<00:00, 88.2MB/s]
92%|#########2| 157M/170M [00:01<00:00, 84.1MB/s]
97%|#########7| 166M/170M [00:01<00:00, 80.4MB/s]
100%|##########| 170M/170M [00:01<00:00, 86.7MB/s]
注意
本节仅适用于对快速结果感兴趣的 CPU 用户。仅当您对小规模实验感兴趣时才使用此选项。请记住,使用任何 GPU,代码都应该运行得相当快。仅从训练/测试数据集中选择前 num_images_to_keep
张图像
#from torch.utils.data import Subset
#num_images_to_keep = 2000
#train_dataset = Subset(train_dataset, range(min(num_images_to_keep, 50_000)))
#test_dataset = Subset(test_dataset, range(min(num_images_to_keep, 10_000)))
#Dataloaders
train_loader = torch.utils.data.DataLoader(train_dataset, batch_size=128, shuffle=True, num_workers=2)
test_loader = torch.utils.data.DataLoader(test_dataset, batch_size=128, shuffle=False, num_workers=2)
定义模型类和实用函数¶
接下来,我们需要定义我们的模型类。此处需要设置几个用户定义的参数。我们使用两种不同的架构,在我们的实验中保持过滤器数量固定,以确保公平比较。两种架构都是卷积神经网络 (CNN),具有不同数量的卷积层,这些卷积层充当特征提取器,后跟一个具有 10 个类别的分类器。学生的过滤器和神经元数量较少。
# Deeper neural network class to be used as teacher:
class DeepNN(nn.Module):
def __init__(self, num_classes=10):
super(DeepNN, self).__init__()
self.features = nn.Sequential(
nn.Conv2d(3, 128, kernel_size=3, padding=1),
nn.ReLU(),
nn.Conv2d(128, 64, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
nn.Conv2d(64, 64, kernel_size=3, padding=1),
nn.ReLU(),
nn.Conv2d(64, 32, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
)
self.classifier = nn.Sequential(
nn.Linear(2048, 512),
nn.ReLU(),
nn.Dropout(0.1),
nn.Linear(512, num_classes)
)
def forward(self, x):
x = self.features(x)
x = torch.flatten(x, 1)
x = self.classifier(x)
return x
# Lightweight neural network class to be used as student:
class LightNN(nn.Module):
def __init__(self, num_classes=10):
super(LightNN, self).__init__()
self.features = nn.Sequential(
nn.Conv2d(3, 16, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
nn.Conv2d(16, 16, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
)
self.classifier = nn.Sequential(
nn.Linear(1024, 256),
nn.ReLU(),
nn.Dropout(0.1),
nn.Linear(256, num_classes)
)
def forward(self, x):
x = self.features(x)
x = torch.flatten(x, 1)
x = self.classifier(x)
return x
我们使用 2 个函数来帮助我们生成和评估原始分类任务的结果。一个函数称为 train
,它接受以下参数
model
:要通过此函数训练(更新其权重)的模型实例。train_loader
:我们上面定义了我们的train_loader
,其工作是将数据馈送到模型中。epochs
:我们循环遍历数据集的次数。learning_rate
:学习率决定了我们朝收敛迈进的步幅有多大。步幅太大或太小都可能有害。device
:确定运行工作负载的设备。可以是 CPU 或 GPU,具体取决于可用性。
我们的测试函数类似,但它将使用 test_loader
调用,以从测试集中加载图像。
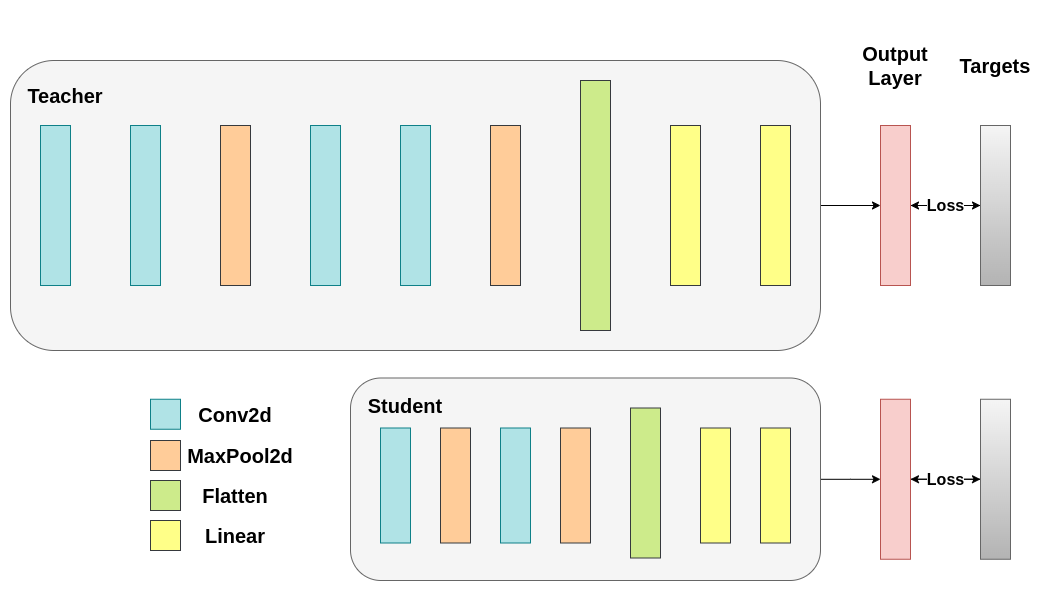
使用交叉熵训练两个网络。学生将用作基线:¶
def train(model, train_loader, epochs, learning_rate, device):
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=learning_rate)
model.train()
for epoch in range(epochs):
running_loss = 0.0
for inputs, labels in train_loader:
# inputs: A collection of batch_size images
# labels: A vector of dimensionality batch_size with integers denoting class of each image
inputs, labels = inputs.to(device), labels.to(device)
optimizer.zero_grad()
outputs = model(inputs)
# outputs: Output of the network for the collection of images. A tensor of dimensionality batch_size x num_classes
# labels: The actual labels of the images. Vector of dimensionality batch_size
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
print(f"Epoch {epoch+1}/{epochs}, Loss: {running_loss / len(train_loader)}")
def test(model, test_loader, device):
model.to(device)
model.eval()
correct = 0
total = 0
with torch.no_grad():
for inputs, labels in test_loader:
inputs, labels = inputs.to(device), labels.to(device)
outputs = model(inputs)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
accuracy = 100 * correct / total
print(f"Test Accuracy: {accuracy:.2f}%")
return accuracy
交叉熵运行¶
为了实现可重复性,我们需要设置 torch 手动种子。我们使用不同的方法训练网络,因此为了公平地比较它们,最好使用相同的权重初始化网络。首先使用交叉熵训练教师网络
torch.manual_seed(42)
nn_deep = DeepNN(num_classes=10).to(device)
train(nn_deep, train_loader, epochs=10, learning_rate=0.001, device=device)
test_accuracy_deep = test(nn_deep, test_loader, device)
# Instantiate the lightweight network:
torch.manual_seed(42)
nn_light = LightNN(num_classes=10).to(device)
Epoch 1/10, Loss: 1.329837580318646
Epoch 2/10, Loss: 0.8678176775003028
Epoch 3/10, Loss: 0.6815747665932111
Epoch 4/10, Loss: 0.5343365599127377
Epoch 5/10, Loss: 0.41786423847650933
Epoch 6/10, Loss: 0.3018103416847146
Epoch 7/10, Loss: 0.22530553787184493
Epoch 8/10, Loss: 0.16732249605228833
Epoch 9/10, Loss: 0.14114774030911953
Epoch 10/10, Loss: 0.11767084830824066
Test Accuracy: 75.98%
我们实例化一个更轻量级的网络模型来比较它们的性能。反向传播对权重初始化很敏感,因此我们需要确保这两个网络具有完全相同的初始化。
torch.manual_seed(42)
new_nn_light = LightNN(num_classes=10).to(device)
为了确保我们创建了第一个网络的副本,我们检查其第一层的范数。如果匹配,那么我们可以安全地得出结论,网络确实是相同的。
# Print the norm of the first layer of the initial lightweight model
print("Norm of 1st layer of nn_light:", torch.norm(nn_light.features[0].weight).item())
# Print the norm of the first layer of the new lightweight model
print("Norm of 1st layer of new_nn_light:", torch.norm(new_nn_light.features[0].weight).item())
Norm of 1st layer of nn_light: 2.327361822128296
Norm of 1st layer of new_nn_light: 2.327361822128296
打印每个模型中的参数总数
total_params_deep = "{:,}".format(sum(p.numel() for p in nn_deep.parameters()))
print(f"DeepNN parameters: {total_params_deep}")
total_params_light = "{:,}".format(sum(p.numel() for p in nn_light.parameters()))
print(f"LightNN parameters: {total_params_light}")
DeepNN parameters: 1,186,986
LightNN parameters: 267,738
使用交叉熵损失训练和测试轻量级网络
train(nn_light, train_loader, epochs=10, learning_rate=0.001, device=device)
test_accuracy_light_ce = test(nn_light, test_loader, device)
Epoch 1/10, Loss: 1.4699091780216187
Epoch 2/10, Loss: 1.1620798132303731
Epoch 3/10, Loss: 1.0295765078281198
Epoch 4/10, Loss: 0.9262913492939356
Epoch 5/10, Loss: 0.8496292234991517
Epoch 6/10, Loss: 0.7809841055089556
Epoch 7/10, Loss: 0.7163250502722952
Epoch 8/10, Loss: 0.6582439961030965
Epoch 9/10, Loss: 0.6072786463343579
Epoch 10/10, Loss: 0.5558338365743837
Test Accuracy: 70.65%
正如我们所看到的,基于测试准确率,我们现在可以将要用作教师的更深层网络与我们假定的学生轻量级网络进行比较。到目前为止,我们的学生尚未干预教师,因此这种性能是由学生自己实现的。到目前为止的指标可以用以下几行代码查看
print(f"Teacher accuracy: {test_accuracy_deep:.2f}%")
print(f"Student accuracy: {test_accuracy_light_ce:.2f}%")
Teacher accuracy: 75.98%
Student accuracy: 70.65%
知识蒸馏运行¶
现在,让我们尝试通过合并教师来提高学生网络的测试准确率。知识蒸馏是一种直接的技术来实现这一点,它基于以下事实:两个网络都输出我们类别的概率分布。因此,这两个网络共享相同数量的输出神经元。该方法的工作原理是将额外的损失纳入传统的交叉熵损失中,该损失基于教师网络的 softmax 输出。假设是,经过适当训练的教师网络的输出激活携带额外的知识,学生网络可以在训练期间利用这些知识。原始工作表明,利用软目标中较小概率的比率可以帮助实现深度神经网络的潜在目标,即在数据上创建相似性结构,其中相似的对象映射得更近。例如,在 CIFAR-10 中,卡车可能会被误认为是汽车或飞机(如果存在车轮),但不太可能被误认为是狗。因此,有理由假设有价值的信息不仅存在于经过适当训练的模型的最高预测中,而且存在于整个输出分布中。然而,仅交叉熵不足以充分利用此信息,因为非预测类别的激活往往非常小,以至于传播的梯度不会有意义地改变权重来构建这种理想的向量空间。
当我们继续定义引入师生动态的第一个辅助函数时,我们需要包含一些额外的参数
T
:温度控制输出分布的平滑度。较大的T
会导致更平滑的分布,因此较小的概率会获得更大的提升。soft_target_loss_weight
:分配给我们要包含的额外目标的权重。ce_loss_weight
:分配给交叉熵的权重。调整这些权重会将网络推向优化任一目标。
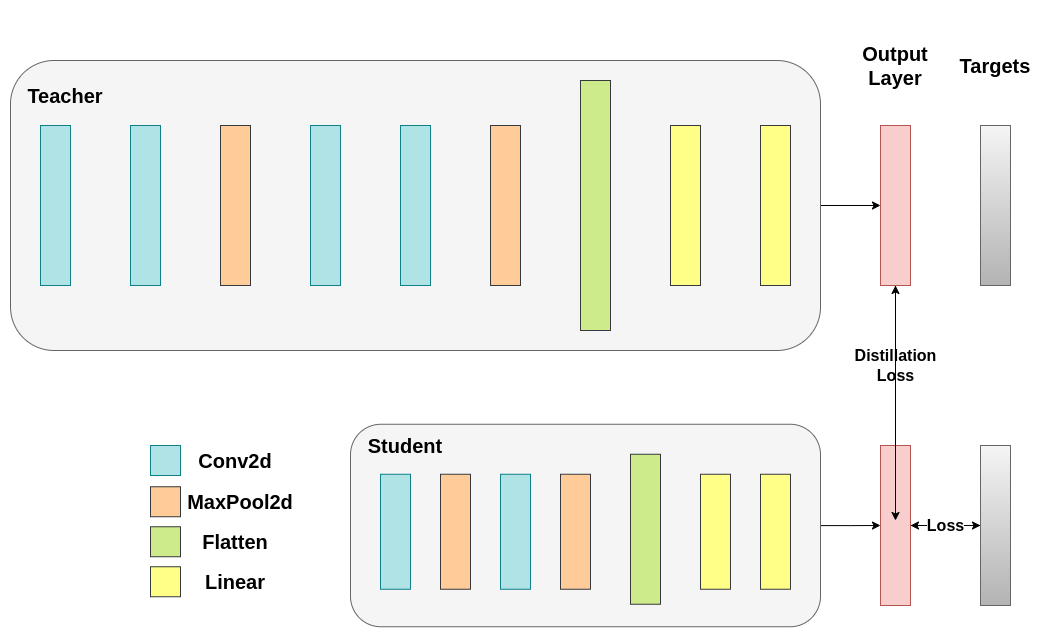
蒸馏损失是从网络的 logits 计算得出的。它仅将梯度返回给学生:¶
def train_knowledge_distillation(teacher, student, train_loader, epochs, learning_rate, T, soft_target_loss_weight, ce_loss_weight, device):
ce_loss = nn.CrossEntropyLoss()
optimizer = optim.Adam(student.parameters(), lr=learning_rate)
teacher.eval() # Teacher set to evaluation mode
student.train() # Student to train mode
for epoch in range(epochs):
running_loss = 0.0
for inputs, labels in train_loader:
inputs, labels = inputs.to(device), labels.to(device)
optimizer.zero_grad()
# Forward pass with the teacher model - do not save gradients here as we do not change the teacher's weights
with torch.no_grad():
teacher_logits = teacher(inputs)
# Forward pass with the student model
student_logits = student(inputs)
#Soften the student logits by applying softmax first and log() second
soft_targets = nn.functional.softmax(teacher_logits / T, dim=-1)
soft_prob = nn.functional.log_softmax(student_logits / T, dim=-1)
# Calculate the soft targets loss. Scaled by T**2 as suggested by the authors of the paper "Distilling the knowledge in a neural network"
soft_targets_loss = torch.sum(soft_targets * (soft_targets.log() - soft_prob)) / soft_prob.size()[0] * (T**2)
# Calculate the true label loss
label_loss = ce_loss(student_logits, labels)
# Weighted sum of the two losses
loss = soft_target_loss_weight * soft_targets_loss + ce_loss_weight * label_loss
loss.backward()
optimizer.step()
running_loss += loss.item()
print(f"Epoch {epoch+1}/{epochs}, Loss: {running_loss / len(train_loader)}")
# Apply ``train_knowledge_distillation`` with a temperature of 2. Arbitrarily set the weights to 0.75 for CE and 0.25 for distillation loss.
train_knowledge_distillation(teacher=nn_deep, student=new_nn_light, train_loader=train_loader, epochs=10, learning_rate=0.001, T=2, soft_target_loss_weight=0.25, ce_loss_weight=0.75, device=device)
test_accuracy_light_ce_and_kd = test(new_nn_light, test_loader, device)
# Compare the student test accuracy with and without the teacher, after distillation
print(f"Teacher accuracy: {test_accuracy_deep:.2f}%")
print(f"Student accuracy without teacher: {test_accuracy_light_ce:.2f}%")
print(f"Student accuracy with CE + KD: {test_accuracy_light_ce_and_kd:.2f}%")
Epoch 1/10, Loss: 2.3993419985027264
Epoch 2/10, Loss: 1.8816983077837073
Epoch 3/10, Loss: 1.660545251863387
Epoch 4/10, Loss: 1.5025935484015422
Epoch 5/10, Loss: 1.377400197641319
Epoch 6/10, Loss: 1.2623111995894585
Epoch 7/10, Loss: 1.1663545374675175
Epoch 8/10, Loss: 1.0826026804916693
Epoch 9/10, Loss: 1.0063069915527578
Epoch 10/10, Loss: 0.9397082798316351
Test Accuracy: 70.49%
Teacher accuracy: 75.98%
Student accuracy without teacher: 70.65%
Student accuracy with CE + KD: 70.49%
余弦损失最小化运行¶
随意调整温度参数(控制 softmax 函数的柔和度)和损失系数。在神经网络中,很容易将额外的损失函数包含到主要目标中,以实现更好的泛化等目标。让我们尝试为学生包含一个目标,但现在让我们关注他们的隐藏状态,而不是他们的输出层。我们的目标是通过包含一个朴素的损失函数来传达来自教师表示的信息给学生,该函数的最小化意味着随后传递给分类器的展平向量在损失减少时变得更加相似。当然,教师不会更新其权重,因此最小化仅取决于学生的权重。这种方法背后的原理是,我们假设教师模型具有更好的内部表示,如果没有外部干预,学生不太可能实现这种内部表示,因此我们人为地推动学生模仿教师的内部表示。然而,这最终是否会帮助学生并不直接,因为推动轻量级网络达到这一点可能是一件好事,假设我们已经找到了一种导致更好测试准确率的内部表示,但它也可能是有害的,因为网络具有不同的架构,并且学生没有与教师相同的学习能力。换句话说,没有理由让这两个向量(学生和教师的向量)按分量匹配。学生可以达到教师内部表示的排列,并且它同样有效。尽管如此,我们仍然可以运行一个快速实验来找出这种方法的影响。我们将使用 CosineEmbeddingLoss
,它由以下公式给出

CosineEmbeddingLoss 的公式¶
显然,我们首先需要解决一件事。当我们对输出层应用蒸馏时,我们提到两个网络具有相同数量的神经元,等于类别的数量。然而,对于卷积层之后的层,情况并非如此。在这里,教师在最终卷积层展平后具有比学生更多的神经元。我们的损失函数接受两个维度相等的向量作为输入,因此我们需要以某种方式匹配它们。我们将通过在教师的卷积层之后包含一个平均池化层来解决这个问题,以将其维度减小到与学生相同的维度。
为了继续,我们将修改我们的模型类,或者创建新的模型类。现在,前向函数不仅返回网络的 logits,还返回卷积层之后的展平隐藏表示。我们为修改后的教师包含前面提到的池化。
class ModifiedDeepNNCosine(nn.Module):
def __init__(self, num_classes=10):
super(ModifiedDeepNNCosine, self).__init__()
self.features = nn.Sequential(
nn.Conv2d(3, 128, kernel_size=3, padding=1),
nn.ReLU(),
nn.Conv2d(128, 64, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
nn.Conv2d(64, 64, kernel_size=3, padding=1),
nn.ReLU(),
nn.Conv2d(64, 32, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
)
self.classifier = nn.Sequential(
nn.Linear(2048, 512),
nn.ReLU(),
nn.Dropout(0.1),
nn.Linear(512, num_classes)
)
def forward(self, x):
x = self.features(x)
flattened_conv_output = torch.flatten(x, 1)
x = self.classifier(flattened_conv_output)
flattened_conv_output_after_pooling = torch.nn.functional.avg_pool1d(flattened_conv_output, 2)
return x, flattened_conv_output_after_pooling
# Create a similar student class where we return a tuple. We do not apply pooling after flattening.
class ModifiedLightNNCosine(nn.Module):
def __init__(self, num_classes=10):
super(ModifiedLightNNCosine, self).__init__()
self.features = nn.Sequential(
nn.Conv2d(3, 16, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
nn.Conv2d(16, 16, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
)
self.classifier = nn.Sequential(
nn.Linear(1024, 256),
nn.ReLU(),
nn.Dropout(0.1),
nn.Linear(256, num_classes)
)
def forward(self, x):
x = self.features(x)
flattened_conv_output = torch.flatten(x, 1)
x = self.classifier(flattened_conv_output)
return x, flattened_conv_output
# We do not have to train the modified deep network from scratch of course, we just load its weights from the trained instance
modified_nn_deep = ModifiedDeepNNCosine(num_classes=10).to(device)
modified_nn_deep.load_state_dict(nn_deep.state_dict())
# Once again ensure the norm of the first layer is the same for both networks
print("Norm of 1st layer for deep_nn:", torch.norm(nn_deep.features[0].weight).item())
print("Norm of 1st layer for modified_deep_nn:", torch.norm(modified_nn_deep.features[0].weight).item())
# Initialize a modified lightweight network with the same seed as our other lightweight instances. This will be trained from scratch to examine the effectiveness of cosine loss minimization.
torch.manual_seed(42)
modified_nn_light = ModifiedLightNNCosine(num_classes=10).to(device)
print("Norm of 1st layer:", torch.norm(modified_nn_light.features[0].weight).item())
Norm of 1st layer for deep_nn: 7.516133785247803
Norm of 1st layer for modified_deep_nn: 7.516133785247803
Norm of 1st layer: 2.327361822128296
当然,我们需要更改训练循环,因为现在模型返回一个元组 (logits, hidden_representation)
。使用示例输入张量,我们可以打印它们的形状。
# Create a sample input tensor
sample_input = torch.randn(128, 3, 32, 32).to(device) # Batch size: 128, Filters: 3, Image size: 32x32
# Pass the input through the student
logits, hidden_representation = modified_nn_light(sample_input)
# Print the shapes of the tensors
print("Student logits shape:", logits.shape) # batch_size x total_classes
print("Student hidden representation shape:", hidden_representation.shape) # batch_size x hidden_representation_size
# Pass the input through the teacher
logits, hidden_representation = modified_nn_deep(sample_input)
# Print the shapes of the tensors
print("Teacher logits shape:", logits.shape) # batch_size x total_classes
print("Teacher hidden representation shape:", hidden_representation.shape) # batch_size x hidden_representation_size
Student logits shape: torch.Size([128, 10])
Student hidden representation shape: torch.Size([128, 1024])
Teacher logits shape: torch.Size([128, 10])
Teacher hidden representation shape: torch.Size([128, 1024])
在我们的例子中,hidden_representation_size
是 1024
。这是学生最终卷积层的展平特征图,如您所见,它是其分类器的输入。对于教师来说,它也是 1024
,因为我们通过来自 2048
的 avg_pool1d
使其如此。此处应用的损失仅影响损失计算之前学生的权重。换句话说,它不会影响学生的分类器。修改后的训练循环如下
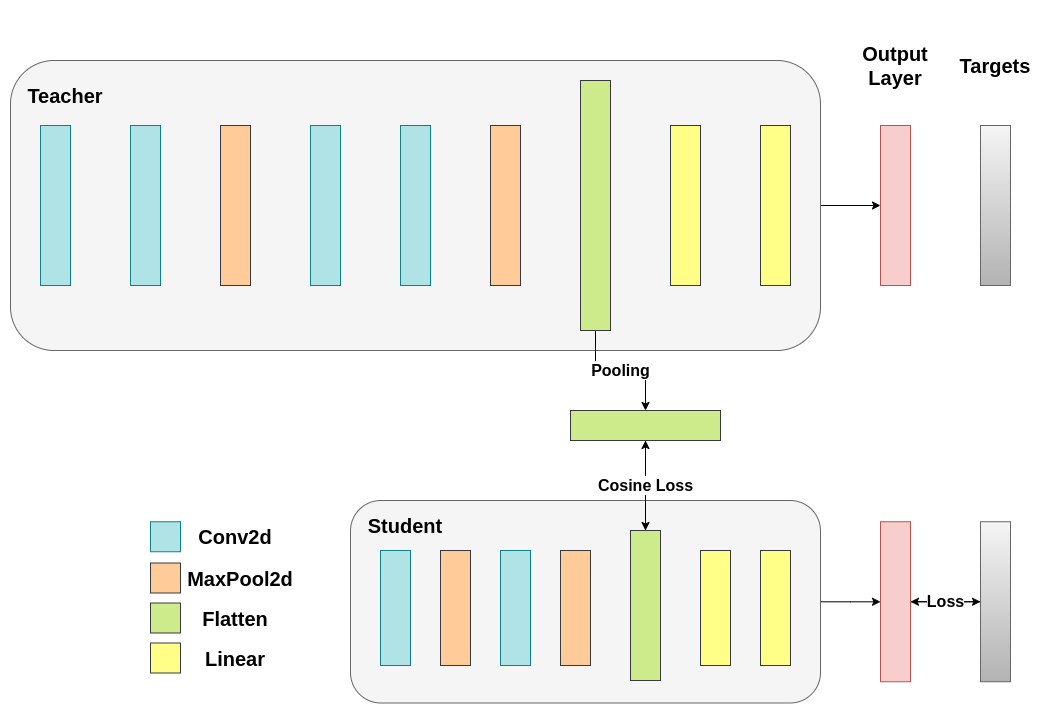
在余弦损失最小化中,我们希望通过将梯度返回给学生来最大化两个表示的余弦相似度:¶
def train_cosine_loss(teacher, student, train_loader, epochs, learning_rate, hidden_rep_loss_weight, ce_loss_weight, device):
ce_loss = nn.CrossEntropyLoss()
cosine_loss = nn.CosineEmbeddingLoss()
optimizer = optim.Adam(student.parameters(), lr=learning_rate)
teacher.to(device)
student.to(device)
teacher.eval() # Teacher set to evaluation mode
student.train() # Student to train mode
for epoch in range(epochs):
running_loss = 0.0
for inputs, labels in train_loader:
inputs, labels = inputs.to(device), labels.to(device)
optimizer.zero_grad()
# Forward pass with the teacher model and keep only the hidden representation
with torch.no_grad():
_, teacher_hidden_representation = teacher(inputs)
# Forward pass with the student model
student_logits, student_hidden_representation = student(inputs)
# Calculate the cosine loss. Target is a vector of ones. From the loss formula above we can see that is the case where loss minimization leads to cosine similarity increase.
hidden_rep_loss = cosine_loss(student_hidden_representation, teacher_hidden_representation, target=torch.ones(inputs.size(0)).to(device))
# Calculate the true label loss
label_loss = ce_loss(student_logits, labels)
# Weighted sum of the two losses
loss = hidden_rep_loss_weight * hidden_rep_loss + ce_loss_weight * label_loss
loss.backward()
optimizer.step()
running_loss += loss.item()
print(f"Epoch {epoch+1}/{epochs}, Loss: {running_loss / len(train_loader)}")
我们需要出于相同的原因修改我们的测试函数。在这里,我们忽略模型返回的隐藏表示。
def test_multiple_outputs(model, test_loader, device):
model.to(device)
model.eval()
correct = 0
total = 0
with torch.no_grad():
for inputs, labels in test_loader:
inputs, labels = inputs.to(device), labels.to(device)
outputs, _ = model(inputs) # Disregard the second tensor of the tuple
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
accuracy = 100 * correct / total
print(f"Test Accuracy: {accuracy:.2f}%")
return accuracy
在这种情况下,我们可以轻松地在同一函数中同时包含知识蒸馏和余弦损失最小化。在师生范式中,组合方法以实现更好的性能是很常见的。现在,我们可以运行一个简单的训练-测试会话。
# Train and test the lightweight network with cross entropy loss
train_cosine_loss(teacher=modified_nn_deep, student=modified_nn_light, train_loader=train_loader, epochs=10, learning_rate=0.001, hidden_rep_loss_weight=0.25, ce_loss_weight=0.75, device=device)
test_accuracy_light_ce_and_cosine_loss = test_multiple_outputs(modified_nn_light, test_loader, device)
Epoch 1/10, Loss: 1.305193693741508
Epoch 2/10, Loss: 1.0703616274897094
Epoch 3/10, Loss: 0.9700422599492475
Epoch 4/10, Loss: 0.8967577328767313
Epoch 5/10, Loss: 0.842951060560963
Epoch 6/10, Loss: 0.7986717939071948
Epoch 7/10, Loss: 0.7563943977246199
Epoch 8/10, Loss: 0.7195081037023793
Epoch 9/10, Loss: 0.6844526155830344
Epoch 10/10, Loss: 0.6541258117274555
Test Accuracy: 70.12%
中间回归器运行¶
我们的朴素最小化并不能保证更好的结果,原因有几个,其中之一是向量的维度。余弦相似度通常比高维度向量的欧几里得距离效果更好,但我们正在处理每个向量具有 1024 个分量的向量,因此更难以提取有意义的相似性。此外,正如我们所提到的,理论不支持推动教师和学生的隐藏表示的匹配。没有充分的理由说明我们应该以这些向量的 1:1 匹配为目标。我们将提供一个最终的训练干预示例,方法是包含一个称为回归器的额外网络。目标是首先提取教师在卷积层之后的特征图,然后提取学生在卷积层之后的特征图,最后尝试匹配这些图。然而,这一次,我们将在网络之间引入一个回归器,以促进匹配过程。回归器将是可训练的,并且理想情况下会比我们朴素的余弦损失最小化方案做得更好。其主要工作是匹配这些特征图的维度,以便我们可以正确定义教师和学生之间的损失函数。定义这样的损失函数提供了一个教学“路径”,这基本上是一个反向传播梯度的流程,该流程将更改学生的权重。关注原始网络中每个分类器之前的卷积层的输出,我们具有以下形状
# Pass the sample input only from the convolutional feature extractor
convolutional_fe_output_student = nn_light.features(sample_input)
convolutional_fe_output_teacher = nn_deep.features(sample_input)
# Print their shapes
print("Student's feature extractor output shape: ", convolutional_fe_output_student.shape)
print("Teacher's feature extractor output shape: ", convolutional_fe_output_teacher.shape)
Student's feature extractor output shape: torch.Size([128, 16, 8, 8])
Teacher's feature extractor output shape: torch.Size([128, 32, 8, 8])
教师有 32 个过滤器,学生有 16 个过滤器。我们将包含一个可训练层,该层将学生的特征图转换为教师特征图的形状。在实践中,我们修改轻量级类以返回中间回归器之后的隐藏状态,该回归器匹配卷积特征图和教师类的大小,以返回最终卷积层的输出,而无需池化或展平。
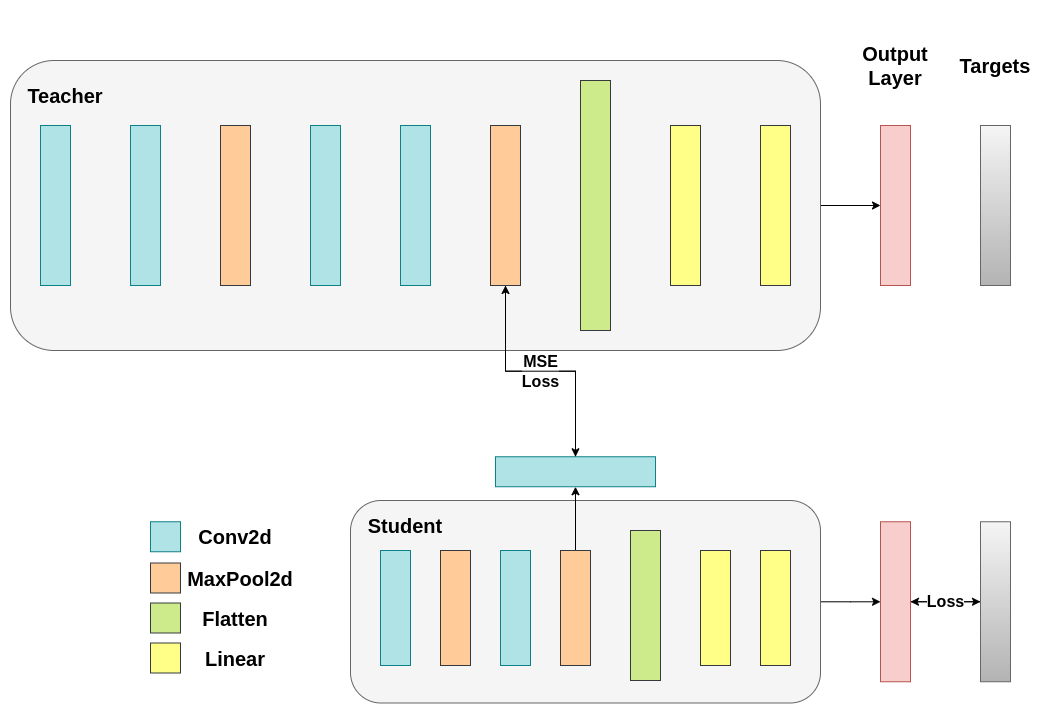
可训练层匹配中间张量的形状,并且正确定义了均方误差 (MSE):¶
class ModifiedDeepNNRegressor(nn.Module):
def __init__(self, num_classes=10):
super(ModifiedDeepNNRegressor, self).__init__()
self.features = nn.Sequential(
nn.Conv2d(3, 128, kernel_size=3, padding=1),
nn.ReLU(),
nn.Conv2d(128, 64, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
nn.Conv2d(64, 64, kernel_size=3, padding=1),
nn.ReLU(),
nn.Conv2d(64, 32, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
)
self.classifier = nn.Sequential(
nn.Linear(2048, 512),
nn.ReLU(),
nn.Dropout(0.1),
nn.Linear(512, num_classes)
)
def forward(self, x):
x = self.features(x)
conv_feature_map = x
x = torch.flatten(x, 1)
x = self.classifier(x)
return x, conv_feature_map
class ModifiedLightNNRegressor(nn.Module):
def __init__(self, num_classes=10):
super(ModifiedLightNNRegressor, self).__init__()
self.features = nn.Sequential(
nn.Conv2d(3, 16, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
nn.Conv2d(16, 16, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
)
# Include an extra regressor (in our case linear)
self.regressor = nn.Sequential(
nn.Conv2d(16, 32, kernel_size=3, padding=1)
)
self.classifier = nn.Sequential(
nn.Linear(1024, 256),
nn.ReLU(),
nn.Dropout(0.1),
nn.Linear(256, num_classes)
)
def forward(self, x):
x = self.features(x)
regressor_output = self.regressor(x)
x = torch.flatten(x, 1)
x = self.classifier(x)
return x, regressor_output
之后,我们必须再次更新我们的训练循环。这一次,我们提取学生的回归器输出、教师的特征图,我们计算这些张量上的 MSE
(它们具有完全相同的形状,因此已正确定义),并且除了分类任务的常规交叉熵损失之外,我们还基于该损失反向传播梯度。
def train_mse_loss(teacher, student, train_loader, epochs, learning_rate, feature_map_weight, ce_loss_weight, device):
ce_loss = nn.CrossEntropyLoss()
mse_loss = nn.MSELoss()
optimizer = optim.Adam(student.parameters(), lr=learning_rate)
teacher.to(device)
student.to(device)
teacher.eval() # Teacher set to evaluation mode
student.train() # Student to train mode
for epoch in range(epochs):
running_loss = 0.0
for inputs, labels in train_loader:
inputs, labels = inputs.to(device), labels.to(device)
optimizer.zero_grad()
# Again ignore teacher logits
with torch.no_grad():
_, teacher_feature_map = teacher(inputs)
# Forward pass with the student model
student_logits, regressor_feature_map = student(inputs)
# Calculate the loss
hidden_rep_loss = mse_loss(regressor_feature_map, teacher_feature_map)
# Calculate the true label loss
label_loss = ce_loss(student_logits, labels)
# Weighted sum of the two losses
loss = feature_map_weight * hidden_rep_loss + ce_loss_weight * label_loss
loss.backward()
optimizer.step()
running_loss += loss.item()
print(f"Epoch {epoch+1}/{epochs}, Loss: {running_loss / len(train_loader)}")
# Notice how our test function remains the same here with the one we used in our previous case. We only care about the actual outputs because we measure accuracy.
# Initialize a ModifiedLightNNRegressor
torch.manual_seed(42)
modified_nn_light_reg = ModifiedLightNNRegressor(num_classes=10).to(device)
# We do not have to train the modified deep network from scratch of course, we just load its weights from the trained instance
modified_nn_deep_reg = ModifiedDeepNNRegressor(num_classes=10).to(device)
modified_nn_deep_reg.load_state_dict(nn_deep.state_dict())
# Train and test once again
train_mse_loss(teacher=modified_nn_deep_reg, student=modified_nn_light_reg, train_loader=train_loader, epochs=10, learning_rate=0.001, feature_map_weight=0.25, ce_loss_weight=0.75, device=device)
test_accuracy_light_ce_and_mse_loss = test_multiple_outputs(modified_nn_light_reg, test_loader, device)
Epoch 1/10, Loss: 1.7066363266971716
Epoch 2/10, Loss: 1.3309815390335629
Epoch 3/10, Loss: 1.188617759196045
Epoch 4/10, Loss: 1.0923469258696221
Epoch 5/10, Loss: 1.0123476643696465
Epoch 6/10, Loss: 0.9512547259135624
Epoch 7/10, Loss: 0.8982603129218606
Epoch 8/10, Loss: 0.8485838961418327
Epoch 9/10, Loss: 0.8041575365054333
Epoch 10/10, Loss: 0.7658210701649756
Test Accuracy: 70.61%
预计最终方法将比 CosineLoss
效果更好,因为现在我们在教师和学生之间允许使用可训练层,当涉及到学习时,这为学生提供了一些回旋余地,而不是推动学生复制教师的表示。包含额外网络是基于提示的蒸馏背后的思想。
print(f"Teacher accuracy: {test_accuracy_deep:.2f}%")
print(f"Student accuracy without teacher: {test_accuracy_light_ce:.2f}%")
print(f"Student accuracy with CE + KD: {test_accuracy_light_ce_and_kd:.2f}%")
print(f"Student accuracy with CE + CosineLoss: {test_accuracy_light_ce_and_cosine_loss:.2f}%")
print(f"Student accuracy with CE + RegressorMSE: {test_accuracy_light_ce_and_mse_loss:.2f}%")
Teacher accuracy: 75.98%
Student accuracy without teacher: 70.65%
Student accuracy with CE + KD: 70.49%
Student accuracy with CE + CosineLoss: 70.12%
Student accuracy with CE + RegressorMSE: 70.61%
结论¶
以上所有方法都不会增加网络的参数数量或推理时间,因此性能的提高是以在训练期间计算梯度的少量成本为代价的。在 ML 应用程序中,我们主要关心推理时间,因为训练发生在模型部署之前。如果我们的轻量级模型对于部署来说仍然太重,我们可以应用不同的想法,例如训练后量化。额外的损失可以应用于许多任务,而不仅仅是分类,您可以试验诸如系数、温度或神经元数量之类的量。随意调整上面教程中的任何数字,但请记住,如果您更改神经元/过滤器的数量,则很可能会发生形状不匹配。
有关更多信息,请参阅
脚本的总运行时间: ( 8 分钟 2.544 秒)