注意
点击这里下载完整的示例代码
TorchVision 对象检测微调教程
创建于:2023 年 12 月 14 日 | 最后更新:2024 年 6 月 11 日 | 最后验证:2024 年 11 月 05 日
在本教程中,我们将对预训练的 Mask R-CNN 模型在 Penn-Fudan 行人检测和分割数据库上进行微调。它包含 170 张图像和 345 个行人实例,我们将使用它来说明如何使用 torchvision 中的新功能,以便在自定义数据集上训练对象检测和实例分割模型。
注意
本教程仅适用于 torchvision 版本 >=0.16 或 nightly 版本。如果您使用的是 torchvision<=0.15,请遵循此教程。
定义数据集
用于训练对象检测、实例分割和人体关键点检测的参考脚本允许轻松支持添加新的自定义数据集。数据集应继承自标准的 torch.utils.data.Dataset
类,并实现 __len__
和 __getitem__
。
我们要求的唯一特殊性是数据集 __getitem__
应返回一个元组
image:形状为
[3, H, W]
的torchvision.tv_tensors.Image
、纯张量或大小为(H, W)
的 PIL 图像target:包含以下字段的字典
boxes
,形状为[N, 4]
的torchvision.tv_tensors.BoundingBoxes
:格式为[x0, y0, x1, y1]
的N
个边界框的坐标,范围从0
到W
和0
到H
labels
,形状为[N]
的整数torch.Tensor
:每个边界框的标签。0
始终代表背景类。image_id
,int:图像标识符。它在数据集中的所有图像之间应该是唯一的,并在评估期间使用area
,形状为[N]
的浮点数torch.Tensor
:边界框的面积。这在 COCO 指标评估期间使用,以区分小、中、大框之间的指标分数。iscrowd
,形状为[N]
的 uint8torch.Tensor
:iscrowd=True
的实例在评估期间将被忽略。(可选)
masks
,形状为[N, H, W]
的torchvision.tv_tensors.Mask
:每个对象的分割掩码
如果您的数据集符合上述要求,那么它将适用于参考脚本中的训练和评估代码。评估代码将使用来自 pycocotools
的脚本,可以使用 pip install pycocotools
安装。
注意
对于 Windows,请从 gautamchitnis 安装 pycocotools
,命令如下
pip install git+https://github.com/gautamchitnis/cocoapi.git@cocodataset-master#subdirectory=PythonAPI
关于 labels
的一点说明。模型将类别 0
视为背景。如果您的数据集不包含背景类,则您的 labels
中不应包含 0
。例如,假设您只有两个类别,猫 和 狗,您可以定义 1
(不是 0
)来表示 猫,2
来表示 狗。因此,例如,如果其中一张图像同时包含这两个类别,则您的 labels
张量应如下所示:[1, 2]
。
此外,如果您想在训练期间使用纵横比分组(以便每个批次仅包含具有相似纵横比的图像),那么建议您也实现一个 get_height_and_width
方法,该方法返回图像的高度和宽度。如果未提供此方法,我们将通过 __getitem__
查询数据集的所有元素,这将图像加载到内存中,速度比提供自定义方法慢。
为 PennFudan 编写自定义数据集
让我们为 PennFudan 数据集编写一个数据集。首先,让我们下载数据集并解压 zip 文件
wget https://www.cis.upenn.edu/~jshi/ped_html/PennFudanPed.zip -P data
cd data && unzip PennFudanPed.zip
我们有以下文件夹结构
PennFudanPed/
PedMasks/
FudanPed00001_mask.png
FudanPed00002_mask.png
FudanPed00003_mask.png
FudanPed00004_mask.png
...
PNGImages/
FudanPed00001.png
FudanPed00002.png
FudanPed00003.png
FudanPed00004.png
这是一个图像和分割掩码对的示例
import matplotlib.pyplot as plt
from torchvision.io import read_image
image = read_image("data/PennFudanPed/PNGImages/FudanPed00046.png")
mask = read_image("data/PennFudanPed/PedMasks/FudanPed00046_mask.png")
plt.figure(figsize=(16, 8))
plt.subplot(121)
plt.title("Image")
plt.imshow(image.permute(1, 2, 0))
plt.subplot(122)
plt.title("Mask")
plt.imshow(mask.permute(1, 2, 0))
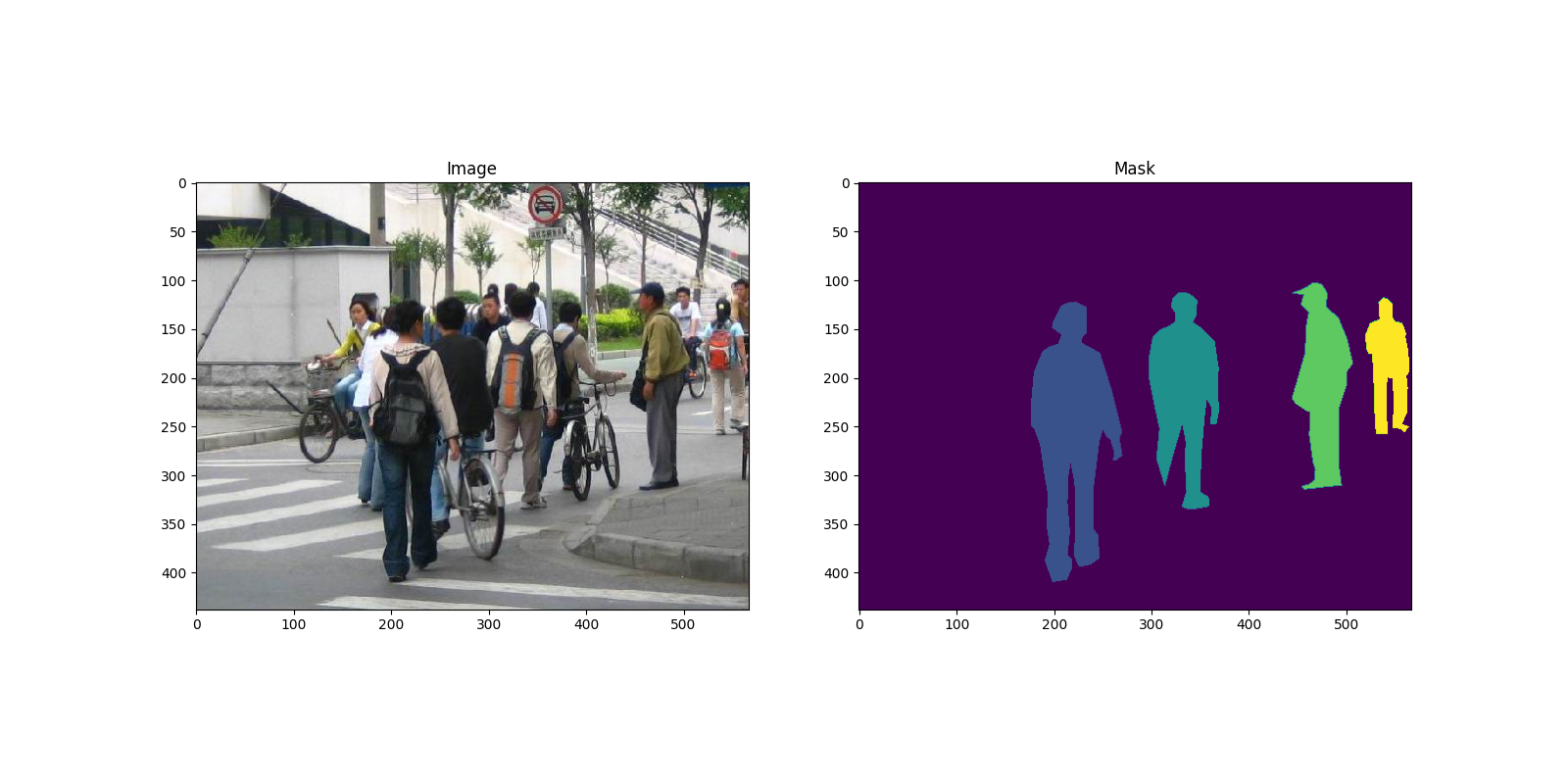
<matplotlib.image.AxesImage object at 0x7f36917736a0>
因此,每张图像都有一个对应的分割掩码,其中每种颜色对应一个不同的实例。让我们为此数据集编写一个 torch.utils.data.Dataset
类。在下面的代码中,我们将图像、边界框和掩码包装到 torchvision.tv_tensors.TVTensor
类中,以便我们能够为给定的对象检测和分割任务应用 torchvision 内置的变换(新的 Transforms API)。即,图像张量将被 torchvision.tv_tensors.Image
包装,边界框将被 torchvision.tv_tensors.BoundingBoxes
包装,掩码将被 torchvision.tv_tensors.Mask
包装。由于 torchvision.tv_tensors.TVTensor
是 torch.Tensor
子类,因此包装的对象也是张量,并继承了普通的 torch.Tensor
API。有关 torchvision tv_tensors
的更多信息,请参阅 此文档。
import os
import torch
from torchvision.io import read_image
from torchvision.ops.boxes import masks_to_boxes
from torchvision import tv_tensors
from torchvision.transforms.v2 import functional as F
class PennFudanDataset(torch.utils.data.Dataset):
def __init__(self, root, transforms):
self.root = root
self.transforms = transforms
# load all image files, sorting them to
# ensure that they are aligned
self.imgs = list(sorted(os.listdir(os.path.join(root, "PNGImages"))))
self.masks = list(sorted(os.listdir(os.path.join(root, "PedMasks"))))
def __getitem__(self, idx):
# load images and masks
img_path = os.path.join(self.root, "PNGImages", self.imgs[idx])
mask_path = os.path.join(self.root, "PedMasks", self.masks[idx])
img = read_image(img_path)
mask = read_image(mask_path)
# instances are encoded as different colors
obj_ids = torch.unique(mask)
# first id is the background, so remove it
obj_ids = obj_ids[1:]
num_objs = len(obj_ids)
# split the color-encoded mask into a set
# of binary masks
masks = (mask == obj_ids[:, None, None]).to(dtype=torch.uint8)
# get bounding box coordinates for each mask
boxes = masks_to_boxes(masks)
# there is only one class
labels = torch.ones((num_objs,), dtype=torch.int64)
image_id = idx
area = (boxes[:, 3] - boxes[:, 1]) * (boxes[:, 2] - boxes[:, 0])
# suppose all instances are not crowd
iscrowd = torch.zeros((num_objs,), dtype=torch.int64)
# Wrap sample and targets into torchvision tv_tensors:
img = tv_tensors.Image(img)
target = {}
target["boxes"] = tv_tensors.BoundingBoxes(boxes, format="XYXY", canvas_size=F.get_size(img))
target["masks"] = tv_tensors.Mask(masks)
target["labels"] = labels
target["image_id"] = image_id
target["area"] = area
target["iscrowd"] = iscrowd
if self.transforms is not None:
img, target = self.transforms(img, target)
return img, target
def __len__(self):
return len(self.imgs)
这就是数据集的全部内容。现在让我们定义一个可以对该数据集执行预测的模型。
定义您的模型
在本教程中,我们将使用 Mask R-CNN,它基于 Faster R-CNN 之上。Faster R-CNN 是一个模型,它预测图像中潜在对象的边界框和类别分数。
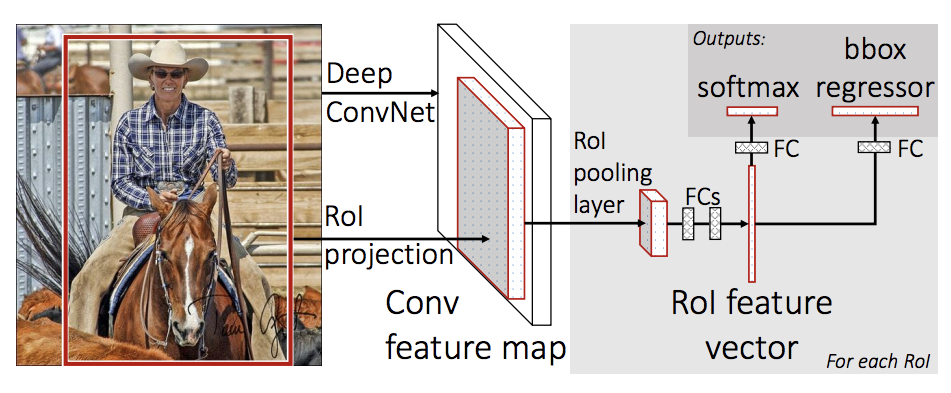
Mask R-CNN 在 Faster R-CNN 中添加了一个额外的分支,该分支还预测每个实例的分割掩码。
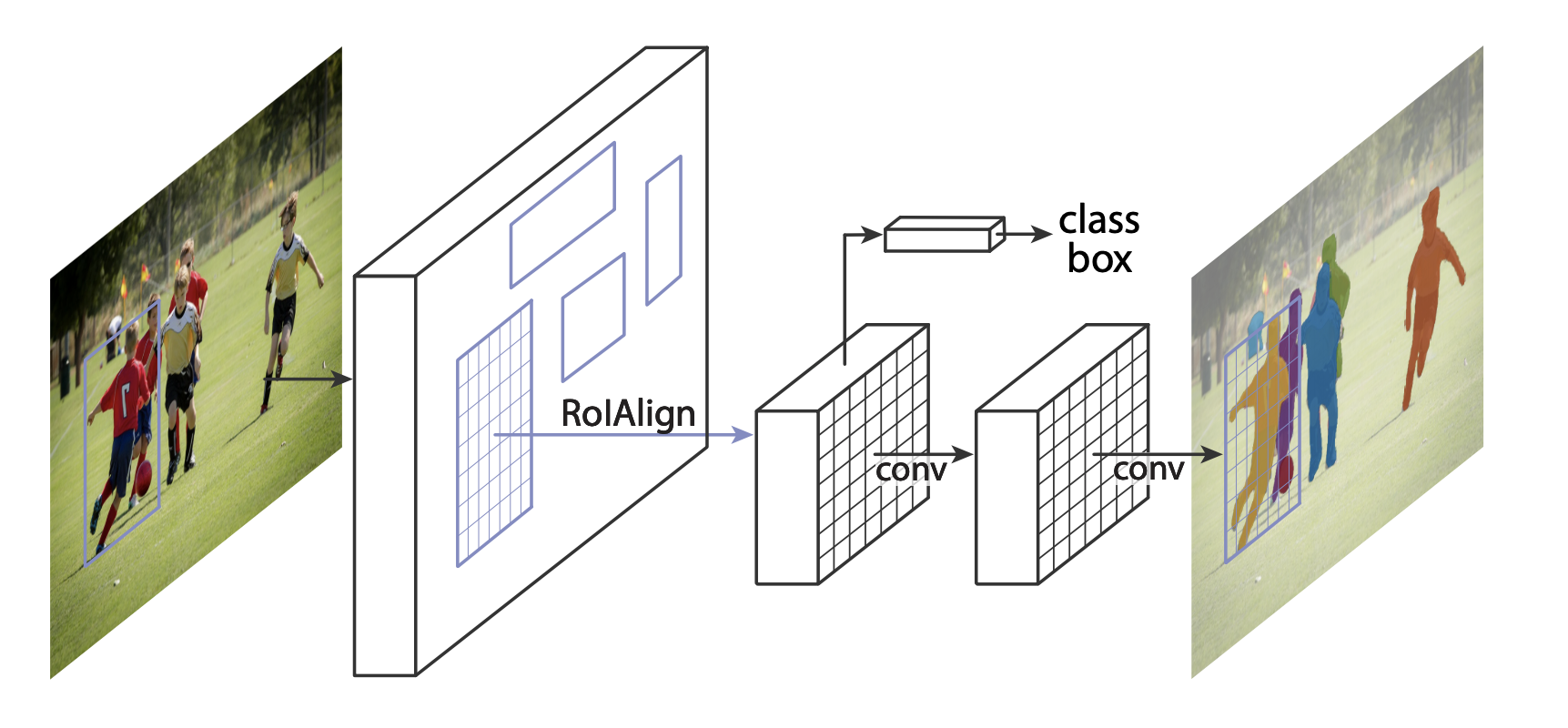
在两种常见情况下,人们可能想要修改 TorchVision Model Zoo 中可用的模型之一。第一种情况是我们想要从预训练模型开始,并仅微调最后一层。另一种情况是我们想要用不同的骨干网络替换模型的骨干网络(例如,为了更快的预测)。
让我们看看在以下部分中我们将如何进行其中一种或另一种操作。
1 - 从预训练模型进行微调
假设您想从在 COCO 上预训练的模型开始,并希望针对您的特定类别对其进行微调。以下是一种可能的方法
import torchvision
from torchvision.models.detection.faster_rcnn import FastRCNNPredictor
# load a model pre-trained on COCO
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(weights="DEFAULT")
# replace the classifier with a new one, that has
# num_classes which is user-defined
num_classes = 2 # 1 class (person) + background
# get number of input features for the classifier
in_features = model.roi_heads.box_predictor.cls_score.in_features
# replace the pre-trained head with a new one
model.roi_heads.box_predictor = FastRCNNPredictor(in_features, num_classes)
Downloading: "https://download.pytorch.org/models/fasterrcnn_resnet50_fpn_coco-258fb6c6.pth" to /var/lib/ci-user/.cache/torch/hub/checkpoints/fasterrcnn_resnet50_fpn_coco-258fb6c6.pth
0%| | 0.00/160M [00:00<?, ?B/s]
27%|##7 | 43.4M/160M [00:00<00:00, 454MB/s]
55%|#####4 | 87.8M/160M [00:00<00:00, 461MB/s]
83%|########2 | 132M/160M [00:00<00:00, 463MB/s]
100%|##########| 160M/160M [00:00<00:00, 462MB/s]
2 - 修改模型以添加不同的骨干网络
import torchvision
from torchvision.models.detection import FasterRCNN
from torchvision.models.detection.rpn import AnchorGenerator
# load a pre-trained model for classification and return
# only the features
backbone = torchvision.models.mobilenet_v2(weights="DEFAULT").features
# ``FasterRCNN`` needs to know the number of
# output channels in a backbone. For mobilenet_v2, it's 1280
# so we need to add it here
backbone.out_channels = 1280
# let's make the RPN generate 5 x 3 anchors per spatial
# location, with 5 different sizes and 3 different aspect
# ratios. We have a Tuple[Tuple[int]] because each feature
# map could potentially have different sizes and
# aspect ratios
anchor_generator = AnchorGenerator(
sizes=((32, 64, 128, 256, 512),),
aspect_ratios=((0.5, 1.0, 2.0),)
)
# let's define what are the feature maps that we will
# use to perform the region of interest cropping, as well as
# the size of the crop after rescaling.
# if your backbone returns a Tensor, featmap_names is expected to
# be [0]. More generally, the backbone should return an
# ``OrderedDict[Tensor]``, and in ``featmap_names`` you can choose which
# feature maps to use.
roi_pooler = torchvision.ops.MultiScaleRoIAlign(
featmap_names=['0'],
output_size=7,
sampling_ratio=2
)
# put the pieces together inside a Faster-RCNN model
model = FasterRCNN(
backbone,
num_classes=2,
rpn_anchor_generator=anchor_generator,
box_roi_pool=roi_pooler
)
Downloading: "https://download.pytorch.org/models/mobilenet_v2-7ebf99e0.pth" to /var/lib/ci-user/.cache/torch/hub/checkpoints/mobilenet_v2-7ebf99e0.pth
0%| | 0.00/13.6M [00:00<?, ?B/s]
100%|##########| 13.6M/13.6M [00:00<00:00, 421MB/s]
用于 PennFudan 数据集的对象检测和实例分割模型
在我们的例子中,我们想从预训练模型进行微调,因为我们的数据集非常小,所以我们将遵循方法 1。
在这里,我们还想计算实例分割掩码,因此我们将使用 Mask R-CNN
import torchvision
from torchvision.models.detection.faster_rcnn import FastRCNNPredictor
from torchvision.models.detection.mask_rcnn import MaskRCNNPredictor
def get_model_instance_segmentation(num_classes):
# load an instance segmentation model pre-trained on COCO
model = torchvision.models.detection.maskrcnn_resnet50_fpn(weights="DEFAULT")
# get number of input features for the classifier
in_features = model.roi_heads.box_predictor.cls_score.in_features
# replace the pre-trained head with a new one
model.roi_heads.box_predictor = FastRCNNPredictor(in_features, num_classes)
# now get the number of input features for the mask classifier
in_features_mask = model.roi_heads.mask_predictor.conv5_mask.in_channels
hidden_layer = 256
# and replace the mask predictor with a new one
model.roi_heads.mask_predictor = MaskRCNNPredictor(
in_features_mask,
hidden_layer,
num_classes
)
return model
就是这样,这将使 model
准备好在您的自定义数据集上进行训练和评估。
将所有内容放在一起
在 references/detection/
中,我们有许多辅助函数来简化检测模型的训练和评估。在这里,我们将使用 references/detection/engine.py
和 references/detection/utils.py
。只需将 references/detection
下的所有内容下载到您的文件夹中,并在此处使用它们。在 Linux 上,如果您有 wget
,您可以使用以下命令下载它们
os.system("wget https://raw.githubusercontent.com/pytorch/vision/main/references/detection/engine.py")
os.system("wget https://raw.githubusercontent.com/pytorch/vision/main/references/detection/utils.py")
os.system("wget https://raw.githubusercontent.com/pytorch/vision/main/references/detection/coco_utils.py")
os.system("wget https://raw.githubusercontent.com/pytorch/vision/main/references/detection/coco_eval.py")
os.system("wget https://raw.githubusercontent.com/pytorch/vision/main/references/detection/transforms.py")
0
自 v0.15.0 torchvision 以来,提供了 新的 Transforms API,可以轻松地为对象检测和分割任务编写数据增强管道。
让我们为数据增强/变换编写一些辅助函数
from torchvision.transforms import v2 as T
def get_transform(train):
transforms = []
if train:
transforms.append(T.RandomHorizontalFlip(0.5))
transforms.append(T.ToDtype(torch.float, scale=True))
transforms.append(T.ToPureTensor())
return T.Compose(transforms)
测试 forward()
方法(可选)
在迭代数据集之前,最好先看看模型在训练和推理时对样本数据的期望。
import utils
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(weights="DEFAULT")
dataset = PennFudanDataset('data/PennFudanPed', get_transform(train=True))
data_loader = torch.utils.data.DataLoader(
dataset,
batch_size=2,
shuffle=True,
collate_fn=utils.collate_fn
)
# For Training
images, targets = next(iter(data_loader))
images = list(image for image in images)
targets = [{k: v for k, v in t.items()} for t in targets]
output = model(images, targets) # Returns losses and detections
print(output)
# For inference
model.eval()
x = [torch.rand(3, 300, 400), torch.rand(3, 500, 400)]
predictions = model(x) # Returns predictions
print(predictions[0])
{'loss_classifier': tensor(0.0808, grad_fn=<NllLossBackward0>), 'loss_box_reg': tensor(0.0284, grad_fn=<DivBackward0>), 'loss_objectness': tensor(0.0186, grad_fn=<BinaryCrossEntropyWithLogitsBackward0>), 'loss_rpn_box_reg': tensor(0.0034, grad_fn=<DivBackward0>)}
{'boxes': tensor([], size=(0, 4), grad_fn=<StackBackward0>), 'labels': tensor([], dtype=torch.int64), 'scores': tensor([], grad_fn=<IndexBackward0>)}
现在让我们编写执行训练和验证的主函数
from engine import train_one_epoch, evaluate
# train on the GPU or on the CPU, if a GPU is not available
device = torch.device('cuda') if torch.cuda.is_available() else torch.device('cpu')
# our dataset has two classes only - background and person
num_classes = 2
# use our dataset and defined transformations
dataset = PennFudanDataset('data/PennFudanPed', get_transform(train=True))
dataset_test = PennFudanDataset('data/PennFudanPed', get_transform(train=False))
# split the dataset in train and test set
indices = torch.randperm(len(dataset)).tolist()
dataset = torch.utils.data.Subset(dataset, indices[:-50])
dataset_test = torch.utils.data.Subset(dataset_test, indices[-50:])
# define training and validation data loaders
data_loader = torch.utils.data.DataLoader(
dataset,
batch_size=2,
shuffle=True,
collate_fn=utils.collate_fn
)
data_loader_test = torch.utils.data.DataLoader(
dataset_test,
batch_size=1,
shuffle=False,
collate_fn=utils.collate_fn
)
# get the model using our helper function
model = get_model_instance_segmentation(num_classes)
# move model to the right device
model.to(device)
# construct an optimizer
params = [p for p in model.parameters() if p.requires_grad]
optimizer = torch.optim.SGD(
params,
lr=0.005,
momentum=0.9,
weight_decay=0.0005
)
# and a learning rate scheduler
lr_scheduler = torch.optim.lr_scheduler.StepLR(
optimizer,
step_size=3,
gamma=0.1
)
# let's train it just for 2 epochs
num_epochs = 2
for epoch in range(num_epochs):
# train for one epoch, printing every 10 iterations
train_one_epoch(model, optimizer, data_loader, device, epoch, print_freq=10)
# update the learning rate
lr_scheduler.step()
# evaluate on the test dataset
evaluate(model, data_loader_test, device=device)
print("That's it!")
Downloading: "https://download.pytorch.org/models/maskrcnn_resnet50_fpn_coco-bf2d0c1e.pth" to /var/lib/ci-user/.cache/torch/hub/checkpoints/maskrcnn_resnet50_fpn_coco-bf2d0c1e.pth
0%| | 0.00/170M [00:00<?, ?B/s]
9%|8 | 14.5M/170M [00:00<00:01, 151MB/s]
17%|#7 | 29.0M/170M [00:00<00:01, 142MB/s]
43%|####2 | 72.4M/170M [00:00<00:00, 280MB/s]
69%|######8 | 116M/170M [00:00<00:00, 350MB/s]
94%|#########4| 160M/170M [00:00<00:00, 389MB/s]
100%|##########| 170M/170M [00:00<00:00, 333MB/s]
/var/lib/workspace/intermediate_source/engine.py:30: FutureWarning:
`torch.cuda.amp.autocast(args...)` is deprecated. Please use `torch.amp.autocast('cuda', args...)` instead.
Epoch: [0] [ 0/60] eta: 0:00:23 lr: 0.000090 loss: 4.9024 (4.9024) loss_classifier: 0.4325 (0.4325) loss_box_reg: 0.1060 (0.1060) loss_mask: 4.3588 (4.3588) loss_objectness: 0.0028 (0.0028) loss_rpn_box_reg: 0.0023 (0.0023) time: 0.3936 data: 0.0141 max mem: 2430
Epoch: [0] [10/60] eta: 0:00:11 lr: 0.000936 loss: 1.7766 (2.7700) loss_classifier: 0.4105 (0.3557) loss_box_reg: 0.3052 (0.2546) loss_mask: 0.9490 (2.1314) loss_objectness: 0.0219 (0.0214) loss_rpn_box_reg: 0.0056 (0.0069) time: 0.2282 data: 0.0157 max mem: 2602
Epoch: [0] [20/60] eta: 0:00:08 lr: 0.001783 loss: 0.8104 (1.7885) loss_classifier: 0.2146 (0.2679) loss_box_reg: 0.2065 (0.2333) loss_mask: 0.3977 (1.2590) loss_objectness: 0.0134 (0.0202) loss_rpn_box_reg: 0.0076 (0.0080) time: 0.2082 data: 0.0159 max mem: 2624
Epoch: [0] [30/60] eta: 0:00:06 lr: 0.002629 loss: 0.6767 (1.4244) loss_classifier: 0.1406 (0.2261) loss_box_reg: 0.2294 (0.2435) loss_mask: 0.2592 (0.9277) loss_objectness: 0.0120 (0.0173) loss_rpn_box_reg: 0.0101 (0.0098) time: 0.2122 data: 0.0169 max mem: 2771
Epoch: [0] [40/60] eta: 0:00:04 lr: 0.003476 loss: 0.5520 (1.2053) loss_classifier: 0.0892 (0.1909) loss_box_reg: 0.2434 (0.2359) loss_mask: 0.2266 (0.7542) loss_objectness: 0.0069 (0.0145) loss_rpn_box_reg: 0.0118 (0.0098) time: 0.2105 data: 0.0169 max mem: 2771
Epoch: [0] [50/60] eta: 0:00:02 lr: 0.004323 loss: 0.3561 (1.0396) loss_classifier: 0.0567 (0.1626) loss_box_reg: 0.1485 (0.2173) loss_mask: 0.1633 (0.6383) loss_objectness: 0.0021 (0.0121) loss_rpn_box_reg: 0.0072 (0.0093) time: 0.2051 data: 0.0158 max mem: 2771
Epoch: [0] [59/60] eta: 0:00:00 lr: 0.005000 loss: 0.3503 (0.9406) loss_classifier: 0.0384 (0.1447) loss_box_reg: 0.1258 (0.2049) loss_mask: 0.1614 (0.5713) loss_objectness: 0.0011 (0.0107) loss_rpn_box_reg: 0.0064 (0.0089) time: 0.2035 data: 0.0150 max mem: 2771
Epoch: [0] Total time: 0:00:12 (0.2108 s / it)
creating index...
index created!
Test: [ 0/50] eta: 0:00:04 model_time: 0.0796 (0.0796) evaluator_time: 0.0073 (0.0073) time: 0.0995 data: 0.0122 max mem: 2771
Test: [49/50] eta: 0:00:00 model_time: 0.0422 (0.0569) evaluator_time: 0.0049 (0.0068) time: 0.0644 data: 0.0096 max mem: 2771
Test: Total time: 0:00:03 (0.0748 s / it)
Averaged stats: model_time: 0.0422 (0.0569) evaluator_time: 0.0049 (0.0068)
Accumulating evaluation results...
DONE (t=0.01s).
Accumulating evaluation results...
DONE (t=0.01s).
IoU metric: bbox
Average Precision (AP) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.634
Average Precision (AP) @[ IoU=0.50 | area= all | maxDets=100 ] = 0.984
Average Precision (AP) @[ IoU=0.75 | area= all | maxDets=100 ] = 0.868
Average Precision (AP) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.288
Average Precision (AP) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.599
Average Precision (AP) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.644
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 1 ] = 0.279
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 10 ] = 0.689
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.689
Average Recall (AR) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.367
Average Recall (AR) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.683
Average Recall (AR) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.698
IoU metric: segm
Average Precision (AP) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.668
Average Precision (AP) @[ IoU=0.50 | area= all | maxDets=100 ] = 0.974
Average Precision (AP) @[ IoU=0.75 | area= all | maxDets=100 ] = 0.782
Average Precision (AP) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.394
Average Precision (AP) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.501
Average Precision (AP) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.682
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 1 ] = 0.292
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 10 ] = 0.724
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.725
Average Recall (AR) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.633
Average Recall (AR) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.683
Average Recall (AR) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.732
Epoch: [1] [ 0/60] eta: 0:00:11 lr: 0.005000 loss: 0.2638 (0.2638) loss_classifier: 0.0195 (0.0195) loss_box_reg: 0.0624 (0.0624) loss_mask: 0.1786 (0.1786) loss_objectness: 0.0001 (0.0001) loss_rpn_box_reg: 0.0032 (0.0032) time: 0.1853 data: 0.0139 max mem: 2771
Epoch: [1] [10/60] eta: 0:00:10 lr: 0.005000 loss: 0.3414 (0.3752) loss_classifier: 0.0520 (0.0535) loss_box_reg: 0.1229 (0.1440) loss_mask: 0.1642 (0.1661) loss_objectness: 0.0029 (0.0034) loss_rpn_box_reg: 0.0082 (0.0082) time: 0.2082 data: 0.0162 max mem: 2771
Epoch: [1] [20/60] eta: 0:00:08 lr: 0.005000 loss: 0.3414 (0.3467) loss_classifier: 0.0380 (0.0449) loss_box_reg: 0.1204 (0.1173) loss_mask: 0.1676 (0.1750) loss_objectness: 0.0016 (0.0024) loss_rpn_box_reg: 0.0069 (0.0071) time: 0.2039 data: 0.0152 max mem: 2771
Epoch: [1] [30/60] eta: 0:00:06 lr: 0.005000 loss: 0.3095 (0.3290) loss_classifier: 0.0367 (0.0450) loss_box_reg: 0.0884 (0.1121) loss_mask: 0.1445 (0.1630) loss_objectness: 0.0009 (0.0022) loss_rpn_box_reg: 0.0044 (0.0068) time: 0.2044 data: 0.0152 max mem: 2771
Epoch: [1] [40/60] eta: 0:00:04 lr: 0.005000 loss: 0.2793 (0.3223) loss_classifier: 0.0429 (0.0439) loss_box_reg: 0.0884 (0.1060) loss_mask: 0.1444 (0.1633) loss_objectness: 0.0011 (0.0021) loss_rpn_box_reg: 0.0052 (0.0070) time: 0.2055 data: 0.0159 max mem: 2771
Epoch: [1] [50/60] eta: 0:00:02 lr: 0.005000 loss: 0.2681 (0.3110) loss_classifier: 0.0314 (0.0420) loss_box_reg: 0.0583 (0.0992) loss_mask: 0.1519 (0.1614) loss_objectness: 0.0009 (0.0019) loss_rpn_box_reg: 0.0045 (0.0065) time: 0.2030 data: 0.0149 max mem: 2771
Epoch: [1] [59/60] eta: 0:00:00 lr: 0.005000 loss: 0.2230 (0.2965) loss_classifier: 0.0314 (0.0406) loss_box_reg: 0.0499 (0.0927) loss_mask: 0.1296 (0.1551) loss_objectness: 0.0005 (0.0018) loss_rpn_box_reg: 0.0031 (0.0062) time: 0.2051 data: 0.0155 max mem: 2771
Epoch: [1] Total time: 0:00:12 (0.2048 s / it)
creating index...
index created!
Test: [ 0/50] eta: 0:00:02 model_time: 0.0442 (0.0442) evaluator_time: 0.0032 (0.0032) time: 0.0600 data: 0.0121 max mem: 2771
Test: [49/50] eta: 0:00:00 model_time: 0.0398 (0.0409) evaluator_time: 0.0028 (0.0040) time: 0.0544 data: 0.0096 max mem: 2771
Test: Total time: 0:00:02 (0.0559 s / it)
Averaged stats: model_time: 0.0398 (0.0409) evaluator_time: 0.0028 (0.0040)
Accumulating evaluation results...
DONE (t=0.01s).
Accumulating evaluation results...
DONE (t=0.01s).
IoU metric: bbox
Average Precision (AP) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.751
Average Precision (AP) @[ IoU=0.50 | area= all | maxDets=100 ] = 0.986
Average Precision (AP) @[ IoU=0.75 | area= all | maxDets=100 ] = 0.929
Average Precision (AP) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.465
Average Precision (AP) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.684
Average Precision (AP) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.765
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 1 ] = 0.320
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 10 ] = 0.798
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.798
Average Recall (AR) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.467
Average Recall (AR) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.767
Average Recall (AR) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.811
IoU metric: segm
Average Precision (AP) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.725
Average Precision (AP) @[ IoU=0.50 | area= all | maxDets=100 ] = 0.972
Average Precision (AP) @[ IoU=0.75 | area= all | maxDets=100 ] = 0.891
Average Precision (AP) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.394
Average Precision (AP) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.577
Average Precision (AP) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.742
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 1 ] = 0.313
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 10 ] = 0.768
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.768
Average Recall (AR) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.533
Average Recall (AR) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.708
Average Recall (AR) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.780
That's it!
因此,经过一个 epoch 的训练后,我们获得了 COCO 风格的 mAP > 50 和 mask mAP 65。
但是预测结果看起来如何?让我们取数据集中的一张图像并验证
import matplotlib.pyplot as plt
from torchvision.utils import draw_bounding_boxes, draw_segmentation_masks
image = read_image("data/PennFudanPed/PNGImages/FudanPed00046.png")
eval_transform = get_transform(train=False)
model.eval()
with torch.no_grad():
x = eval_transform(image)
# convert RGBA -> RGB and move to device
x = x[:3, ...].to(device)
predictions = model([x, ])
pred = predictions[0]
image = (255.0 * (image - image.min()) / (image.max() - image.min())).to(torch.uint8)
image = image[:3, ...]
pred_labels = [f"pedestrian: {score:.3f}" for label, score in zip(pred["labels"], pred["scores"])]
pred_boxes = pred["boxes"].long()
output_image = draw_bounding_boxes(image, pred_boxes, pred_labels, colors="red")
masks = (pred["masks"] > 0.7).squeeze(1)
output_image = draw_segmentation_masks(output_image, masks, alpha=0.5, colors="blue")
plt.figure(figsize=(12, 12))
plt.imshow(output_image.permute(1, 2, 0))
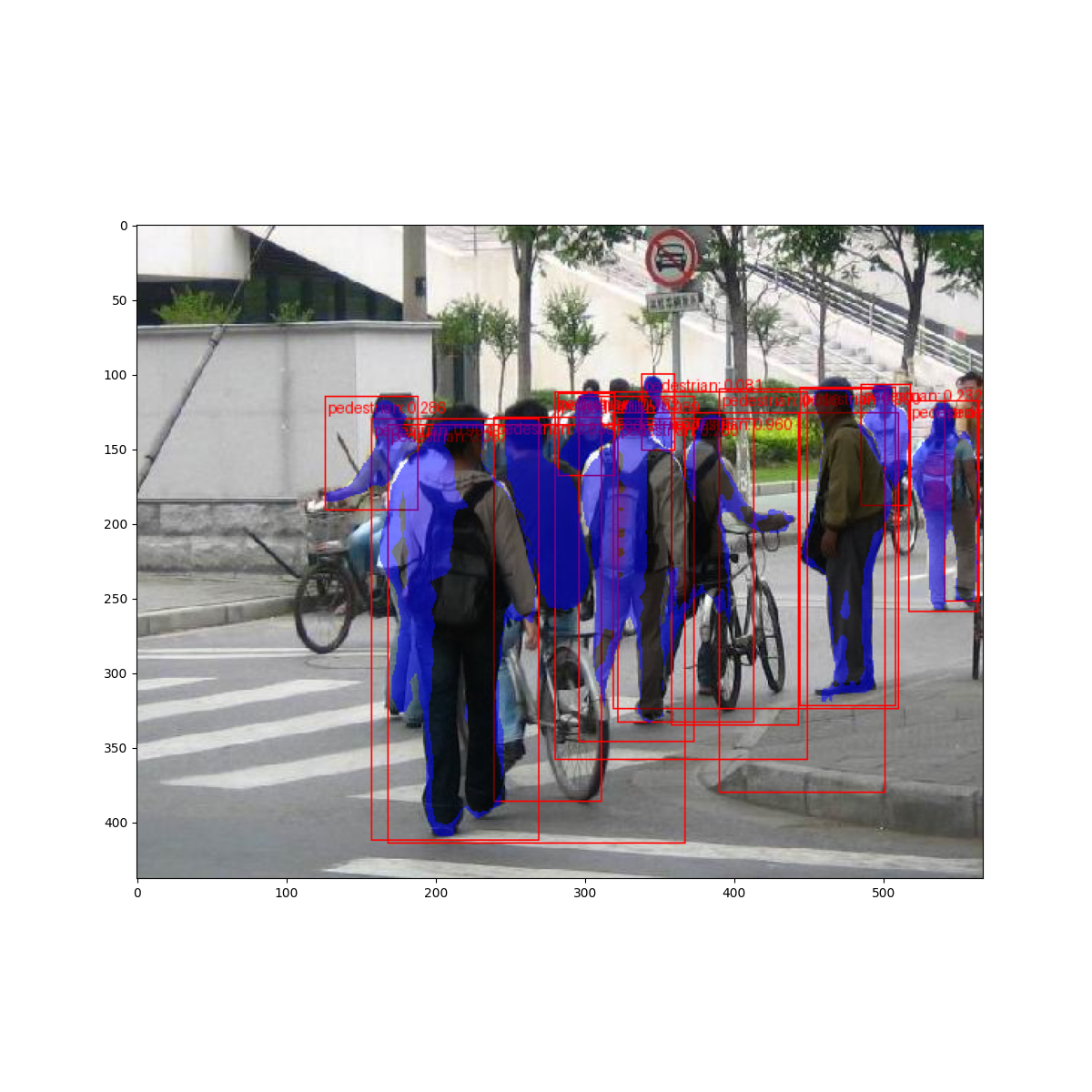
<matplotlib.image.AxesImage object at 0x7f3691de7d00>
结果看起来不错!
总结
在本教程中,您学习了如何为自定义数据集上的对象检测模型创建自己的训练管道。为此,您编写了一个 torch.utils.data.Dataset
类,该类返回图像以及真值框和分割掩码。您还利用了在 COCO train2017 上预训练的 Mask R-CNN 模型,以便在此新数据集上执行迁移学习。
有关更完整的示例,包括多机器/多 GPU 训练,请查看 torchvision 存储库中存在的 references/detection/train.py
。
脚本总运行时间: ( 0 分钟 45.484 秒)