注意
点击此处下载完整示例代码
NLP 从零开始:使用字符级 RNN 生成姓名¶
创建于: 2017 年 3 月 24 日 | 最后更新于: 2024 年 10 月 21 日 | 最后验证于: 2024 年 11 月 5 日
作者: Sean Robertson
本教程是三部分系列教程的一部分
这是我们“NLP 从零开始”系列三部分教程中的第二部分。在第一个教程中,我们使用了 RNN 将姓名分类到其语言来源。这次我们将反过来,从语言生成姓名。
> python sample.py Russian RUS
Rovakov
Uantov
Shavakov
> python sample.py German GER
Gerren
Ereng
Rosher
> python sample.py Spanish SPA
Salla
Parer
Allan
> python sample.py Chinese CHI
Chan
Hang
Iun
我们仍然手工构建了一个带有几个线性层的小型 RNN。主要区别在于,我们不再是在读取所有字母后预测类别,而是输入一个类别并一次输出一个字母。循环预测字符以形成语言(这也可以使用单词或其他更高阶的结构来完成)通常被称为“语言模型”。
推荐阅读
我假设您至少已安装 PyTorch,了解 Python,并理解张量 (Tensors)
使用 PyTorch 进行深度学习:60 分钟速成 了解 PyTorch 的基本用法
通过示例学习 PyTorch 获取广泛而深入的概览
PyTorch for Former Torch Users 如果您是 Lua Torch 用户
了解 RNN 及其工作原理也会有所帮助
循环神经网络的惊人有效性 展示了许多实际示例
理解 LSTM 网络 专门介绍了 LSTM,但对于理解 RNN 总体上也有帮助
我还建议阅读前一个教程:NLP 从零开始:使用字符级 RNN 对姓名进行分类
准备数据¶
注意
从这里下载数据并将其解压到当前目录。
有关此过程的更多详细信息,请参见上一个教程。简而言之,有许多纯文本文件 data/names/[Language].txt
,每行一个姓名。我们将行拆分成数组,将 Unicode 转换为 ASCII,最终得到一个字典 {语言: [姓名 ...]}
。
from io import open
import glob
import os
import unicodedata
import string
all_letters = string.ascii_letters + " .,;'-"
n_letters = len(all_letters) + 1 # Plus EOS marker
def findFiles(path): return glob.glob(path)
# Turn a Unicode string to plain ASCII, thanks to https://stackoverflow.com/a/518232/2809427
def unicodeToAscii(s):
return ''.join(
c for c in unicodedata.normalize('NFD', s)
if unicodedata.category(c) != 'Mn'
and c in all_letters
)
# Read a file and split into lines
def readLines(filename):
with open(filename, encoding='utf-8') as some_file:
return [unicodeToAscii(line.strip()) for line in some_file]
# Build the category_lines dictionary, a list of lines per category
category_lines = {}
all_categories = []
for filename in findFiles('data/names/*.txt'):
category = os.path.splitext(os.path.basename(filename))[0]
all_categories.append(category)
lines = readLines(filename)
category_lines[category] = lines
n_categories = len(all_categories)
if n_categories == 0:
raise RuntimeError('Data not found. Make sure that you downloaded data '
'from https://download.pytorch.org/tutorial/data.zip and extract it to '
'the current directory.')
print('# categories:', n_categories, all_categories)
print(unicodeToAscii("O'Néàl"))
# categories: 18 ['Arabic', 'Chinese', 'Czech', 'Dutch', 'English', 'French', 'German', 'Greek', 'Irish', 'Italian', 'Japanese', 'Korean', 'Polish', 'Portuguese', 'Russian', 'Scottish', 'Spanish', 'Vietnamese']
O'Neal
创建网络¶
这个网络扩展了上一个教程的 RNN,增加了一个用于类别张量 (category tensor) 的额外参数,该参数与其他张量连接在一起。类别张量与字母输入一样,是一个独热 (one-hot) 向量。
我们将把输出解释为下一个字母的概率。在采样时,概率最高的输出字母用作下一个输入字母。
我添加了第二个线性层 o2o
(在组合隐藏层和输出层之后),以赋予它更强大的处理能力。还有一个 Dropout 层,它会以给定的概率 (此处为 0.1) 随机将部分输入归零,通常用于模糊输入以防止过拟合。在这里,我们将其用在网络的末端,有意地增加一些随机性并增加采样多样性。

import torch
import torch.nn as nn
class RNN(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(RNN, self).__init__()
self.hidden_size = hidden_size
self.i2h = nn.Linear(n_categories + input_size + hidden_size, hidden_size)
self.i2o = nn.Linear(n_categories + input_size + hidden_size, output_size)
self.o2o = nn.Linear(hidden_size + output_size, output_size)
self.dropout = nn.Dropout(0.1)
self.softmax = nn.LogSoftmax(dim=1)
def forward(self, category, input, hidden):
input_combined = torch.cat((category, input, hidden), 1)
hidden = self.i2h(input_combined)
output = self.i2o(input_combined)
output_combined = torch.cat((hidden, output), 1)
output = self.o2o(output_combined)
output = self.dropout(output)
output = self.softmax(output)
return output, hidden
def initHidden(self):
return torch.zeros(1, self.hidden_size)
训练¶
准备训练¶
首先,获取随机 (类别, 行) 对的辅助函数
import random
# Random item from a list
def randomChoice(l):
return l[random.randint(0, len(l) - 1)]
# Get a random category and random line from that category
def randomTrainingPair():
category = randomChoice(all_categories)
line = randomChoice(category_lines[category])
return category, line
对于每个时间步(即,训练单词中的每个字母),网络的输入将是 (类别, 当前字母, 隐藏状态)
,输出将是 (下一个字母, 下一个隐藏状态)
。因此,对于每个训练集,我们将需要类别、一组输入字母和一组输出/目标字母。
由于我们在每个时间步从当前字母预测下一个字母,字母对是来自该行的连续字母组 - 例如,对于 "ABCD<EOS>"
,我们将创建 ("A", "B")、("B", "C")、("C", "D")、("D", "EOS")。

类别张量是一个大小为 <1 x n_categories>
的独热张量。训练时,我们在每个时间步将其馈送给网络——这是一个设计选择,它也可以作为初始隐藏状态的一部分或其他策略包含进来。
# One-hot vector for category
def categoryTensor(category):
li = all_categories.index(category)
tensor = torch.zeros(1, n_categories)
tensor[0][li] = 1
return tensor
# One-hot matrix of first to last letters (not including EOS) for input
def inputTensor(line):
tensor = torch.zeros(len(line), 1, n_letters)
for li in range(len(line)):
letter = line[li]
tensor[li][0][all_letters.find(letter)] = 1
return tensor
# ``LongTensor`` of second letter to end (EOS) for target
def targetTensor(line):
letter_indexes = [all_letters.find(line[li]) for li in range(1, len(line))]
letter_indexes.append(n_letters - 1) # EOS
return torch.LongTensor(letter_indexes)
为了训练方便,我们将创建一个 randomTrainingExample
函数,用于获取随机的 (类别, 行) 对,并将其转换为所需的 (类别, 输入, 目标) 张量。
# Make category, input, and target tensors from a random category, line pair
def randomTrainingExample():
category, line = randomTrainingPair()
category_tensor = categoryTensor(category)
input_line_tensor = inputTensor(line)
target_line_tensor = targetTensor(line)
return category_tensor, input_line_tensor, target_line_tensor
训练网络¶
与仅使用最后一个输出的分类不同,我们在每个步骤进行预测,因此我们在每个步骤计算损失。
autograd 的神奇之处在于,您可以简单地将每个步骤的这些损失相加,并在最后调用 backward。
criterion = nn.NLLLoss()
learning_rate = 0.0005
def train(category_tensor, input_line_tensor, target_line_tensor):
target_line_tensor.unsqueeze_(-1)
hidden = rnn.initHidden()
rnn.zero_grad()
loss = torch.Tensor([0]) # you can also just simply use ``loss = 0``
for i in range(input_line_tensor.size(0)):
output, hidden = rnn(category_tensor, input_line_tensor[i], hidden)
l = criterion(output, target_line_tensor[i])
loss += l
loss.backward()
for p in rnn.parameters():
p.data.add_(p.grad.data, alpha=-learning_rate)
return output, loss.item() / input_line_tensor.size(0)
为了跟踪训练所需的时间,我添加了一个 timeSince(timestamp)
函数,该函数返回一个人可读的字符串。
import time
import math
def timeSince(since):
now = time.time()
s = now - since
m = math.floor(s / 60)
s -= m * 60
return '%dm %ds' % (m, s)
训练照常进行——多次调用 train 并等待几分钟,每隔 print_every
个示例打印当前时间和损失,并每隔 plot_every
个示例将平均损失存储在 all_losses
中,以便稍后绘制。
rnn = RNN(n_letters, 128, n_letters)
n_iters = 100000
print_every = 5000
plot_every = 500
all_losses = []
total_loss = 0 # Reset every ``plot_every`` ``iters``
start = time.time()
for iter in range(1, n_iters + 1):
output, loss = train(*randomTrainingExample())
total_loss += loss
if iter % print_every == 0:
print('%s (%d %d%%) %.4f' % (timeSince(start), iter, iter / n_iters * 100, loss))
if iter % plot_every == 0:
all_losses.append(total_loss / plot_every)
total_loss = 0
0m 10s (5000 5%) 2.7686
0m 20s (10000 10%) 2.5023
0m 31s (15000 15%) 3.0934
0m 42s (20000 20%) 2.2183
0m 52s (25000 25%) 2.7072
1m 3s (30000 30%) 2.0118
1m 13s (35000 35%) 2.6332
1m 24s (40000 40%) 2.5150
1m 34s (45000 45%) 2.1207
1m 45s (50000 50%) 2.8377
1m 55s (55000 55%) 2.6050
2m 5s (60000 60%) 2.5402
2m 16s (65000 65%) 2.7429
2m 26s (70000 70%) 1.5367
2m 37s (75000 75%) 0.8677
2m 47s (80000 80%) 3.1882
2m 58s (85000 85%) 1.9431
3m 8s (90000 90%) 2.7269
3m 19s (95000 95%) 3.2194
3m 29s (100000 100%) 1.7136
绘制损失曲线¶
绘制 all_losses 中的历史损失曲线显示了网络的学习情况
import matplotlib.pyplot as plt
plt.figure()
plt.plot(all_losses)
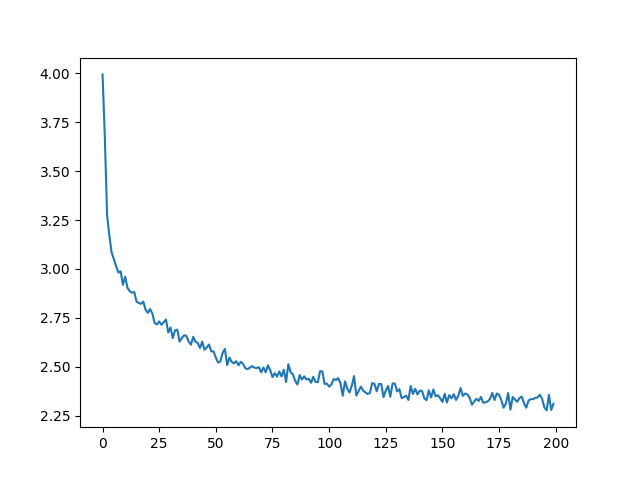
[<matplotlib.lines.Line2D object at 0x7f12a1748f70>]
网络采样¶
要进行采样,我们给网络一个字母并询问下一个字母是什么,将该字母作为下一个输入,重复直到 EOS 标记。
创建输入类别、起始字母和空隐藏状态的张量
创建一个以起始字母开头的字符串
output_name
在最大输出长度内,
将当前字母馈送给网络
从最高概率输出中获取下一个字母,以及下一个隐藏状态
如果字母是 EOS,则停止
如果是一个普通字母,添加到
output_name
并继续
返回最终的姓名
注意
另一种策略是,不必给定起始字母,而是在训练中包含一个“字符串开始”标记,让网络选择自己的起始字母。
max_length = 20
# Sample from a category and starting letter
def sample(category, start_letter='A'):
with torch.no_grad(): # no need to track history in sampling
category_tensor = categoryTensor(category)
input = inputTensor(start_letter)
hidden = rnn.initHidden()
output_name = start_letter
for i in range(max_length):
output, hidden = rnn(category_tensor, input[0], hidden)
topv, topi = output.topk(1)
topi = topi[0][0]
if topi == n_letters - 1:
break
else:
letter = all_letters[topi]
output_name += letter
input = inputTensor(letter)
return output_name
# Get multiple samples from one category and multiple starting letters
def samples(category, start_letters='ABC'):
for start_letter in start_letters:
print(sample(category, start_letter))
samples('Russian', 'RUS')
samples('German', 'GER')
samples('Spanish', 'SPA')
samples('Chinese', 'CHI')
Rovakov
Uakinov
Shantov
Gangeng
Erenger
Ronger
Sarera
Parer
Aras
Chan
Han
Iu
练习¶
尝试使用不同的类别 -> 行数据集,例如
虚构系列 -> 角色姓名
词性 -> 单词
国家 -> 城市
使用“句子开始”标记,以便无需选择起始字母即可进行采样
使用更大和/或形状更好的网络获得更好的结果
尝试使用
nn.LSTM
和nn.GRU
层将多个这样的 RNN 组合成一个更高层次的网络
脚本总运行时间: ( 3 分 29.841 秒)