注意
点击 这里 下载完整示例代码
神经网络¶
可以使用 torch.nn
包构建神经网络。
现在你已经对 autograd
有了初步了解,nn
依赖于 autograd
来定义模型并对其进行微分。一个 nn.Module
包含层,以及一个方法 forward(input)
,它返回 output
。
例如,看看这个对数字图像进行分类的网络
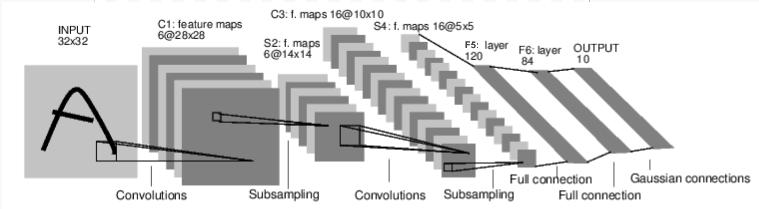
卷积神经网络¶
这是一个简单的前馈网络。它接收输入,将其依次馈送到多个层中,然后最终输出结果。
神经网络的典型训练过程如下
定义具有某些可学习参数(或权重)的神经网络
迭代输入数据集
通过网络处理输入
计算损失(输出与正确结果的距离)
将梯度反向传播到网络参数中
更新网络权重,通常使用一个简单的更新规则:
weight = weight - learning_rate * gradient
定义网络¶
让我们定义这个网络
import torch
import torch.nn as nn
import torch.nn.functional as F
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
# 1 input image channel, 6 output channels, 5x5 square convolution
# kernel
self.conv1 = nn.Conv2d(1, 6, 5)
self.conv2 = nn.Conv2d(6, 16, 5)
# an affine operation: y = Wx + b
self.fc1 = nn.Linear(16 * 5 * 5, 120) # 5*5 from image dimension
self.fc2 = nn.Linear(120, 84)
self.fc3 = nn.Linear(84, 10)
def forward(self, input):
# Convolution layer C1: 1 input image channel, 6 output channels,
# 5x5 square convolution, it uses RELU activation function, and
# outputs a Tensor with size (N, 6, 28, 28), where N is the size of the batch
c1 = F.relu(self.conv1(input))
# Subsampling layer S2: 2x2 grid, purely functional,
# this layer does not have any parameter, and outputs a (N, 6, 14, 14) Tensor
s2 = F.max_pool2d(c1, (2, 2))
# Convolution layer C3: 6 input channels, 16 output channels,
# 5x5 square convolution, it uses RELU activation function, and
# outputs a (N, 16, 10, 10) Tensor
c3 = F.relu(self.conv2(s2))
# Subsampling layer S4: 2x2 grid, purely functional,
# this layer does not have any parameter, and outputs a (N, 16, 5, 5) Tensor
s4 = F.max_pool2d(c3, 2)
# Flatten operation: purely functional, outputs a (N, 400) Tensor
s4 = torch.flatten(s4, 1)
# Fully connected layer F5: (N, 400) Tensor input,
# and outputs a (N, 120) Tensor, it uses RELU activation function
f5 = F.relu(self.fc1(s4))
# Fully connected layer F6: (N, 120) Tensor input,
# and outputs a (N, 84) Tensor, it uses RELU activation function
f6 = F.relu(self.fc2(f5))
# Gaussian layer OUTPUT: (N, 84) Tensor input, and
# outputs a (N, 10) Tensor
output = self.fc3(f6)
return output
net = Net()
print(net)
Net(
(conv1): Conv2d(1, 6, kernel_size=(5, 5), stride=(1, 1))
(conv2): Conv2d(6, 16, kernel_size=(5, 5), stride=(1, 1))
(fc1): Linear(in_features=400, out_features=120, bias=True)
(fc2): Linear(in_features=120, out_features=84, bias=True)
(fc3): Linear(in_features=84, out_features=10, bias=True)
)
您只需要定义 forward
函数,backward
函数(用于计算梯度)会使用 autograd
自动为您定义。您可以在 forward
函数中使用任何张量运算。
net.parameters()
返回模型的可学习参数。
params = list(net.parameters())
print(len(params))
print(params[0].size()) # conv1's .weight
10
torch.Size([6, 1, 5, 5])
让我们尝试一个随机的 32x32 输入。注意:此网络 (LeNet) 的预期输入大小为 32x32。要在 MNIST 数据集上使用此网络,请将数据集中的图像调整为 32x32 大小。
input = torch.randn(1, 1, 32, 32)
out = net(input)
print(out)
tensor([[ 0.1453, -0.0590, -0.0065, 0.0905, 0.0146, -0.0805, -0.1211, -0.0394,
-0.0181, -0.0136]], grad_fn=<AddmmBackward0>)
将所有参数的梯度缓冲区清零并使用随机梯度进行反向传播。
net.zero_grad()
out.backward(torch.randn(1, 10))
注意
torch.nn
仅支持小批量。整个 torch.nn
包仅支持作为样本小批量,而不是单个样本的输入。
例如,nn.Conv2d
将接收一个 4D 张量,其形状为 nSamples x nChannels x Height x Width
。
如果您只有一个样本,只需使用 input.unsqueeze(0)
添加一个假的批次维度。
在继续之前,让我们回顾一下迄今为止您所见过的所有类。
- 回顾
torch.Tensor
- 一个支持backward()
等自动微分运算的多维数组。它还保存着关于张量的梯度。nn.Module
- 神经网络模块。一种将参数封装起来方便的方法,并提供用于将它们移动到 GPU、导出、加载等的帮助器。nn.Parameter
- 一种张量,它在作为属性被分配给Module
时会自动注册为参数。autograd.Function
- 实现自动微分运算的正向和反向定义。每个Tensor
运算至少会创建一个连接到创建了Tensor
的函数的单个Function
节点,并编码其历史记录。
- 到目前为止,我们已经涵盖了
定义神经网络
处理输入并调用反向传播
- 剩下部分
计算损失
更新网络的权重
损失函数¶
损失函数接收 (输出,目标) 对作为输入,并计算一个值来估计输出与目标的距离。
nn 包中包含几种不同的 损失函数。一个简单的损失是:nn.MSELoss
,它计算输出与目标之间的均方误差。
例如
tensor(1.3619, grad_fn=<MseLossBackward0>)
现在,如果您按照 loss
的反向方向进行,使用其 .grad_fn
属性,您将看到一个这样的计算图
input -> conv2d -> relu -> maxpool2d -> conv2d -> relu -> maxpool2d
-> flatten -> linear -> relu -> linear -> relu -> linear
-> MSELoss
-> loss
因此,当我们调用 loss.backward()
时,整个图会针对神经网络参数进行微分,图中所有具有 requires_grad=True
的张量都会在其 .grad
张量中累积梯度。
为了说明,让我们反向跟踪几个步骤
<MseLossBackward0 object at 0x7f45f30f4fd0>
<AddmmBackward0 object at 0x7f45f30f5180>
<AccumulateGrad object at 0x7f45f30f6f80>
反向传播¶
为了反向传播误差,我们只需要 loss.backward()
。但是,您需要清除现有的梯度,否则梯度会累积到现有的梯度中。
现在我们将调用 loss.backward()
,并在反向传播之前和之后查看 conv1 的偏差梯度。
net.zero_grad() # zeroes the gradient buffers of all parameters
print('conv1.bias.grad before backward')
print(net.conv1.bias.grad)
loss.backward()
print('conv1.bias.grad after backward')
print(net.conv1.bias.grad)
conv1.bias.grad before backward
None
conv1.bias.grad after backward
tensor([ 0.0081, -0.0080, -0.0039, 0.0150, 0.0003, -0.0105])
现在,我们已经了解了如何使用损失函数。
稍后阅读
神经网络包包含各种模块和损失函数,它们构成了深度神经网络的构建块。完整的列表及其文档 在此。
剩下的唯一需要学习的是
更新网络的权重
更新权重¶
实践中最简单的更新规则是随机梯度下降 (SGD)
weight = weight - learning_rate * gradient
我们可以使用简单的 Python 代码来实现它
learning_rate = 0.01
for f in net.parameters():
f.data.sub_(f.grad.data * learning_rate)
但是,当您使用神经网络时,您想要使用各种不同的更新规则,例如 SGD、Nesterov-SGD、Adam、RMSProp 等。为了实现这一点,我们构建了一个小包:torch.optim
,它实现了所有这些方法。使用它非常简单
import torch.optim as optim
# create your optimizer
optimizer = optim.SGD(net.parameters(), lr=0.01)
# in your training loop:
optimizer.zero_grad() # zero the gradient buffers
output = net(input)
loss = criterion(output, target)
loss.backward()
optimizer.step() # Does the update
注意
观察如何使用 optimizer.zero_grad()
手动将梯度缓冲区设置为零。这是因为梯度会累积,正如 反向传播 部分所解释的那样。
脚本的总运行时间: ( 0 分钟 0.038 秒)