Inspector API¶
概述¶
Inspector API 提供了一个便捷的接口,用于分析 ETRecord 和 ETDump 的内容,帮助开发者深入了解模型架构和性能统计信息。它构建在 EventBlock 类 数据结构之上,该结构组织了一组 Event,以便轻松访问性能分析事件的详细信息。
用户可以通过多种方式与 Inspector API 进行交互
请参考 e2e 用例文档,了解如何在实际示例中使用这些功能。
Inspector 方法¶
构造函数¶
- executorch.devtools.Inspector.__init__(self, etdump_path=None, etdump_data=None, etrecord=None, source_time_scale=TimeScale.NS, target_time_scale=TimeScale.MS, debug_buffer_path=None, delegate_metadata_parser=None, delegate_time_scale_converter=None, enable_module_hierarchy=False)¶
初始化一个 Inspector 实例,其底层 EventBlocks 使用提供的 ETDump 路径或二进制数据填充,并可选择提供 ETRecord 路径。
- 参数
etdump_path – ETDump 文件路径。必须提供此参数或 etdump_data。
etdump_data – ETDump 二进制数据。必须提供此参数或 etdump_path。
etrecord – 可选的 ETRecord 对象或 ETRecord 文件路径。
source_time_scale – 从运行时检索到的性能数据的时间刻度。运行时中默认的时间 hook 实现返回 NS。
target_time_scale – 用户希望将性能数据转换为的目标时间刻度。默认为 MS。
debug_buffer_path – 调试缓冲区文件路径,包含 ETDump 引用的中间和程序输出的调试数据。
delegate_metadata_parser – 可选函数,用于从 Profiling Event 解析 delegate 元数据。预期函数签名是:(delegate_metadata_list: List[bytes]) -> Union[List[str], Dict[str, Any]]
delegate_time_scale_converter – 可选函数,用于转换 delegate 性能分析数据的时间刻度。如果未提供,则使用 target_time_scale/source_time_scale 的转换比例。
enable_module_hierarchy – 在算子图中启用子模块。默认为 False。
- 返回值
无
示例用法
from executorch.devtools import Inspector
inspector = Inspector(etdump_path="/path/to/etdump.etdp", etrecord="/path/to/etrecord.bin")
to_dataframe¶
- executorch.devtools.Inspector.to_dataframe(self, include_units=True, include_delegate_debug_data=False)¶
- 参数
include_units – 标头是否应包含单位(默认为 true)
include_delegate_debug_data – 是否包含 delegate 调试元数据(默认为 false)
- 返回值
返回一个 pandas DataFrame,其中包含 inspector 中每个 EventBlock 的 Event,每行代表一个 Event。
print_data_tabular¶
- executorch.devtools.Inspector.print_data_tabular(self, file=<sphinx_gallery.gen_rst._LoggingTee object>, include_units=True, include_delegate_debug_data=False)¶
以结构化表格格式显示底层 EventBlocks,每行代表一个 Event。
- 参数
file – 打印到哪个 IO 流。默认为 stdout。如果在 IPython 环境(例如 Jupyter notebook)中则不使用。
include_units – 标头是否应包含单位(默认为 true)
include_delegate_debug_data – 是否包含 delegate 调试元数据(默认为 false)
- 返回值
无
示例用法
inspector.print_data_tabular()
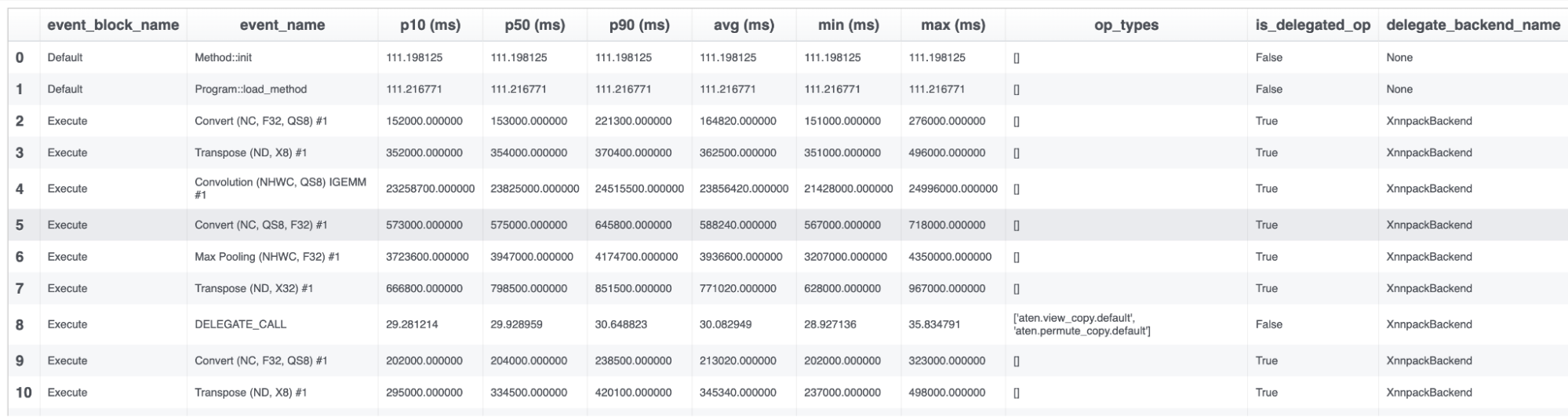
请注意,delegate 性能分析事件的单位是“cycles”。我们正在努力将来提供一种设置不同单位的方法。
find_total_for_module¶
- executorch.devtools.Inspector.find_total_for_module(self, module_name)¶
返回指定模块中所有算子的总平均计算时间。
- 参数
module_name – 要聚合的模块名称。
- 返回值
名称为“module_name”的模块中所有算子的总平均计算时间(以秒为单位)。
示例用法
print(inspector.find_total_for_module("L__self___conv_layer"))
0.002
get_exported_program¶
- executorch.devtools.Inspector.get_exported_program(self, graph=None)¶
ETRecord 的访问助手,默认为返回 Edge Dialect program。
- 参数
graph – 要访问的图的可选名称。如果为 None,则返回 Edge Dialect program。
- 返回值
“graph”的 ExportedProgram 对象。
示例用法
print(inspector.get_exported_program())
ExportedProgram:
class GraphModule(torch.nn.Module):
def forward(self, arg0_1: f32[4, 3, 64, 64]):
# No stacktrace found for following nodes
_param_constant0 = self._param_constant0
_param_constant1 = self._param_constant1
### ... Omit part of the program for documentation readability ... ###
Graph signature: ExportGraphSignature(parameters=[], buffers=[], user_inputs=['arg0_1'], user_outputs=['aten_tan_default'], inputs_to_parameters={}, inputs_to_buffers={}, buffers_to_mutate={}, backward_signature=None, assertion_dep_token=None)
Range constraints: {}
Equality constraints: []
Inspector 属性¶
EventBlock
类¶
通过 Inspector
实例的 event_blocks
属性访问 EventBlock
实例,例如
inspector.event_blocks
- class executorch.devtools.inspector.EventBlock(name, events=<factory>, source_time_scale=TimeScale.NS, target_time_scale=TimeScale.MS, bundled_input_index=None, run_output=None, reference_output=None)[source]¶
一个 EventBlock 包含与从运行时检索到的特定性能分析/调试块相关联的事件集合。每个 EventBlock 代表一种执行模式。例如,模型初始化和加载存在于单个 EventBlock 中。如果存在控制流,每个分支将由一个单独的 EventBlock 表示。
- 参数
name – 性能分析/调试块的名称。
events – 与性能分析/调试块相关联的 Event 列表。
bundled_input_idx – 此 EventBlock 对应的 Bundled Input 的索引。
run_output – 从封装的 Events 中提取的运行输出。
Event
类¶
通过 EventBlock
实例的 events
属性访问 Event
实例。
- class executorch.devtools.inspector.Event(name, perf_data=None, op_types=<factory>, delegate_debug_identifier=None, debug_handles=None, stack_traces=<factory>, module_hierarchy=<factory>, is_delegated_op=None, delegate_backend_name=None, _delegate_debug_metadatas=<factory>, debug_data=<factory>, _instruction_id=None, _delegate_metadata_parser=None, _delegate_time_scale_converter=None)[source]¶
一个 Event 对应一个算子实例,该实例具有从运行时检索到的性能数据和从 ETRecord 获取的其他元数据。
- 参数
name – 性能分析 Event 的名称,如果没有性能分析事件则为空。
perf_data – 与从运行时检索到的事件相关联的性能数据(可用属性:p10, p50, p90, avg, min and max)。
op_type – 与事件对应的算子类型列表。
delegate_debug_identifier – 与 instruction id 结合使用的补充标识符。
debug_handles – 模型图中与此事件相关的调试句柄。
stack_trace – 将每个关联算子的名称映射到其堆栈跟踪的字典。
module_hierarchy – 将每个关联算子的名称映射到其模块层次结构的字典。
is_delegated_op – 事件是否被 delegate。
delegate_backend_name – 此事件被 delegate 到的后端名称。
_delegate_debug_metadatas – 原始 delegate 调试元数据的字符串列表,每个性能分析事件对应一个。如果提供了解析器,则作为 Event.delegate_debug_metadatas 可用;也作为 Event.raw_delegate_debug_metadatas 可用。
debug_data – 包含收集到的中间数据的列表。
_instruction_id – 用于符号化的 Instruction 标识符。
_delegate_metadata_parser – _delegate_debug_metadatas 的可选解析器。
示例用法
for event_block in inspector.event_blocks:
for event in event_block.events:
if event.name == "Method::execute":
print(event.perf_data.raw)
[175.748, 78.678, 70.429, 122.006, 97.495, 67.603, 70.2, 90.139, 66.344, 64.575, 134.135, 93.85, 74.593, 83.929, 75.859, 73.909, 66.461, 72.102, 84.142, 77.774, 70.038, 80.246, 59.134, 68.496, 67.496, 100.491, 81.162, 74.53, 70.709, 77.112, 59.775, 79.674, 67.54, 79.52, 66.753, 70.425, 71.703, 81.373, 72.306, 72.404, 94.497, 77.588, 79.835, 68.597, 71.237, 88.528, 71.884, 74.047, 81.513, 76.116]
CLI¶
在您的终端中执行以下命令以显示数据表。此命令产生的表格输出与调用前面提到的 print_data_tabular 函数相同
python3 -m devtools.inspector.inspector_cli --etdump_path <path_to_etdump> --etrecord_path <path_to_etrecord>
请注意,etrecord_path 参数是可选的。
我们计划将来扩展 CLI 的功能。