后端和委托¶
受众:供应商、后端委托开发人员,他们有兴趣将自己的编译器和硬件集成到 ExecuTorch 中
后端委托是后端处理和执行 PyTorch 程序的入口点,以利用专用后端和硬件的性能和效率优势,同时仍为 PyTorch 用户提供接近 PyTorch 运行时的体验。
后端接口:概述¶
从高级别来看,后端的入口点由两个组件定义
用于表示程序的 IR:**边缘方言**(通过
to_edge
API 生成)后端需要实现的几个接口
提前 (AOT)
程序预处理(例如提前编译、转换、优化…)。
运行时
程序初始化(例如运行时编译)。
程序执行。
(可选)程序销毁(例如释放后端拥有的资源)。
委托后端实现由以下部分组成
提前预处理接口
运行时初始化和执行接口
图表如下所示
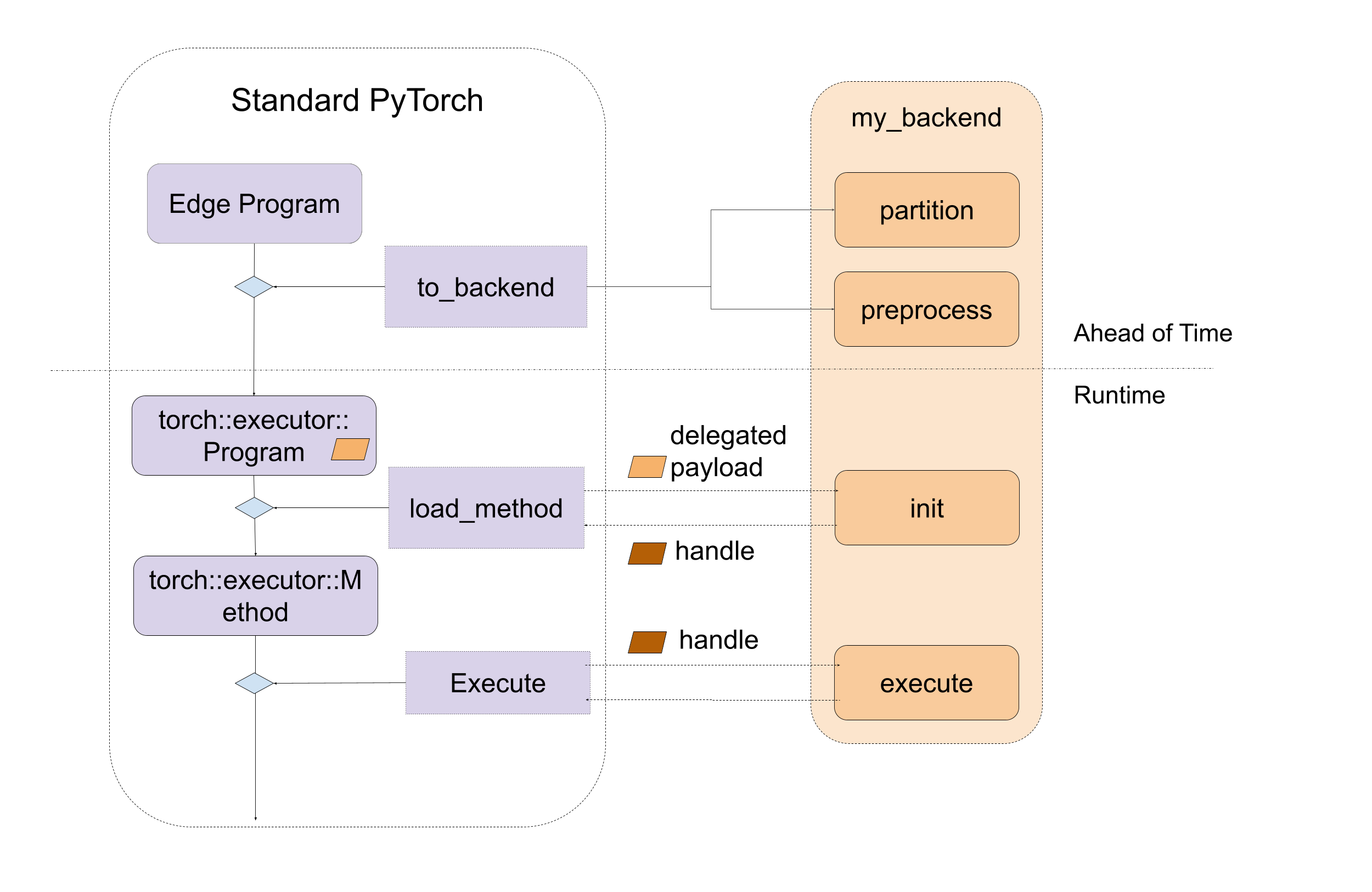
图 1. 后端接口的入口点的高级视图,包括提前和运行时。
后端接口:提前预处理¶
后端主要有两个提前入口点需要实现:partition
和 preprocess
。
partitioner
是后端实现的一种算法,用于标记要降低到后端的节点。 to_backend
API 将应用分区算法并将每个子图(由连接的标记节点组成)降低到目标后端。 每个子图将被发送到后端提供的 preprocess
部分,以编译为二进制 blob。
在分区期间,exported_program
不允许修改程序,它应该为每个节点应用标记。 PartitionResult
包括已标记的导出程序和用于 to_backend
的分区标记字典,用于查找标记并链接到 backend_id
和 compile_spec
def partition(
exported_program: ExportedProgram,
) -> PartitionResult:
在预处理期间,后端将获得一个边缘方言程序、一个编译规范列表(指定编译所需的数值),并期望返回一个已编译的 blob 或包含要在后端运行的所需程序的二进制文件。 在序列化期间,已编译的 blob 将被序列化为 .pte
文件的一部分,并直接加载到设备。 此过程的 API 是
def preprocess(
edge_program: ExportedProgram,
compile_specs: List[CompileSpec],
) -> PreprocessResult:
preprocess 函数的演示实现 在这里。 演示循环遍历 edge_program
的图模块中的节点,并将 add
、mul
和 sin
指令序列化为字符串,该字符串稍后将在运行时被解析和执行。
图表如下所示
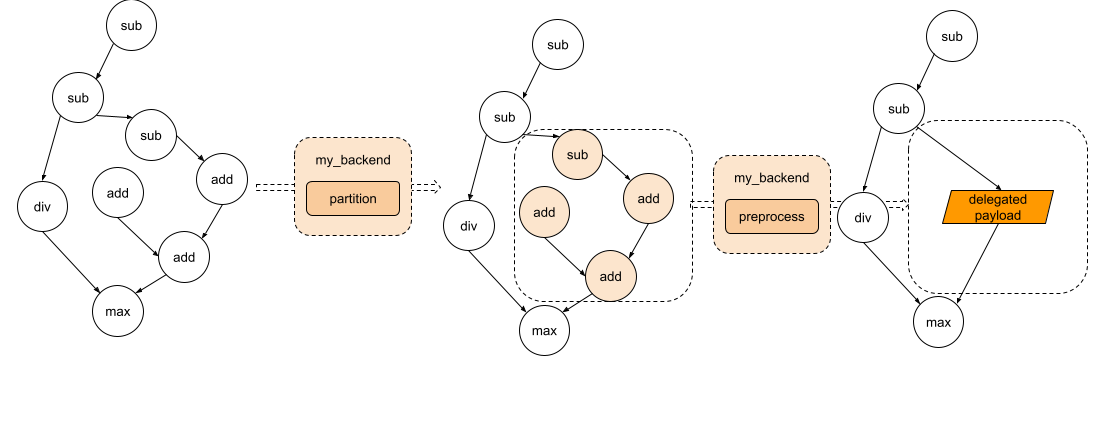
图 2. 图形经过分区,每个子图将被发送到预处理部分。
后端接口:运行时初始化和执行¶
在运行时,来自 preprocess
函数的已编译 blob 将被加载并直接传递到后端的自定义 init
函数。 此函数负责进一步处理已编译的单元,以及执行任何后端初始化。 然后将调用后端的自定义 execute
函数来执行由 init
生成的句柄。 最后,如果某些后端需要销毁,后端可以实现一个 destroy
函数,该函数将在程序超出其生命周期时被调用。
// Runtime check
ET_NODISCARD bool is_available();
// Runtime initialization
ET_NODISCARD virtual Result<DelegateHandle*> init(
BackendInitContext& context,
FreeableBuffer* processed,
ArrayRef<CompileSpec> compile_specs);
// Runtime execution
ET_NODISCARD virtual Error execute(
BackendExecutionContext& context,
DelegateHandle* handle,
EValue** args);
// [optional] Runtime destroy. Destroy the resource held by the backend
virtual void destroy(ET_UNUSED DelegateHandle* handle);
图表如下所示
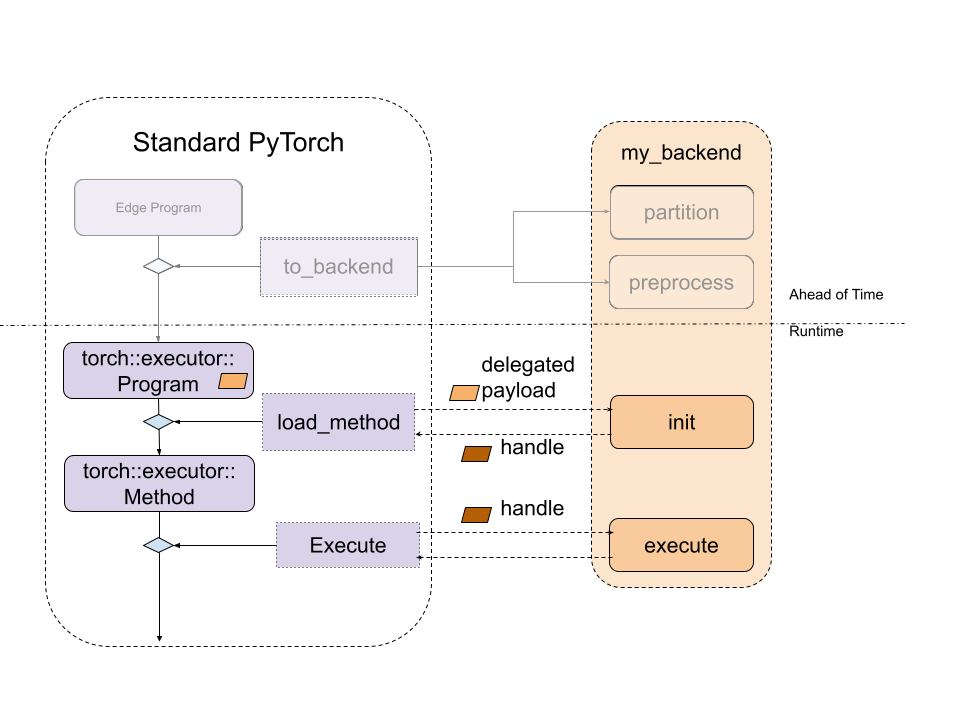
图 3. 标准 ExecuTorch 运行时与后端入口点之间的关系。
为了使后端可用于 ExecuTorch 运行时,必须通过 register_backend
API 注册它。
ET_NODISCARD Error register_backend(const Backend& backend);
静态注册,即在库初始化或加载时注册后端,可以按如下方式实现。
namespace {
auto cls = BackendWithCompiler();
Backend backend{"BackendWithCompilerDemo", &cls};
static auto success_with_compiler = register_backend(backend);
} // namespace
开发者工具集成:可调试性¶
提供一致的调试体验非常重要,无论是针对运行时故障还是性能分析。 ExecuTorch 为此目的采用本地开发者工具,这些工具允许通过调试句柄将程序指令与原始 PyTorch 代码相关联。 你可以阅读更多关于它的信息 在这里.
委托程序或子图对 ExecuTorch 运行时是透明的,表现为一个特殊的 call_delegate
指令,它请求相应的后端处理子图或程序的执行。由于后端委托的透明性,原生开发者工具无法看到委托程序。因此,与非委托对应程序相比,委托执行的调试、功能或性能体验会显著下降。
为了为用户提供一致的调试体验,无论模型是否使用委托,开发者工具提供了一个接口来将委托的(子)图与原始的(子)图关联起来。开发者工具通过调试句柄映射来实现这一点,该映射允许委托生成可以与委托使用的原始(子)图关联的内部句柄。然后在运行时,后端开发者可以使用内部句柄报告错误或性能分析信息,这些信息将使用调试句柄映射映射到原始(子)图。有关更多信息,请参阅 委托调试。
通过利用调试标识符,后端开发者可以将调试作为委托 blob 的一部分嵌入。
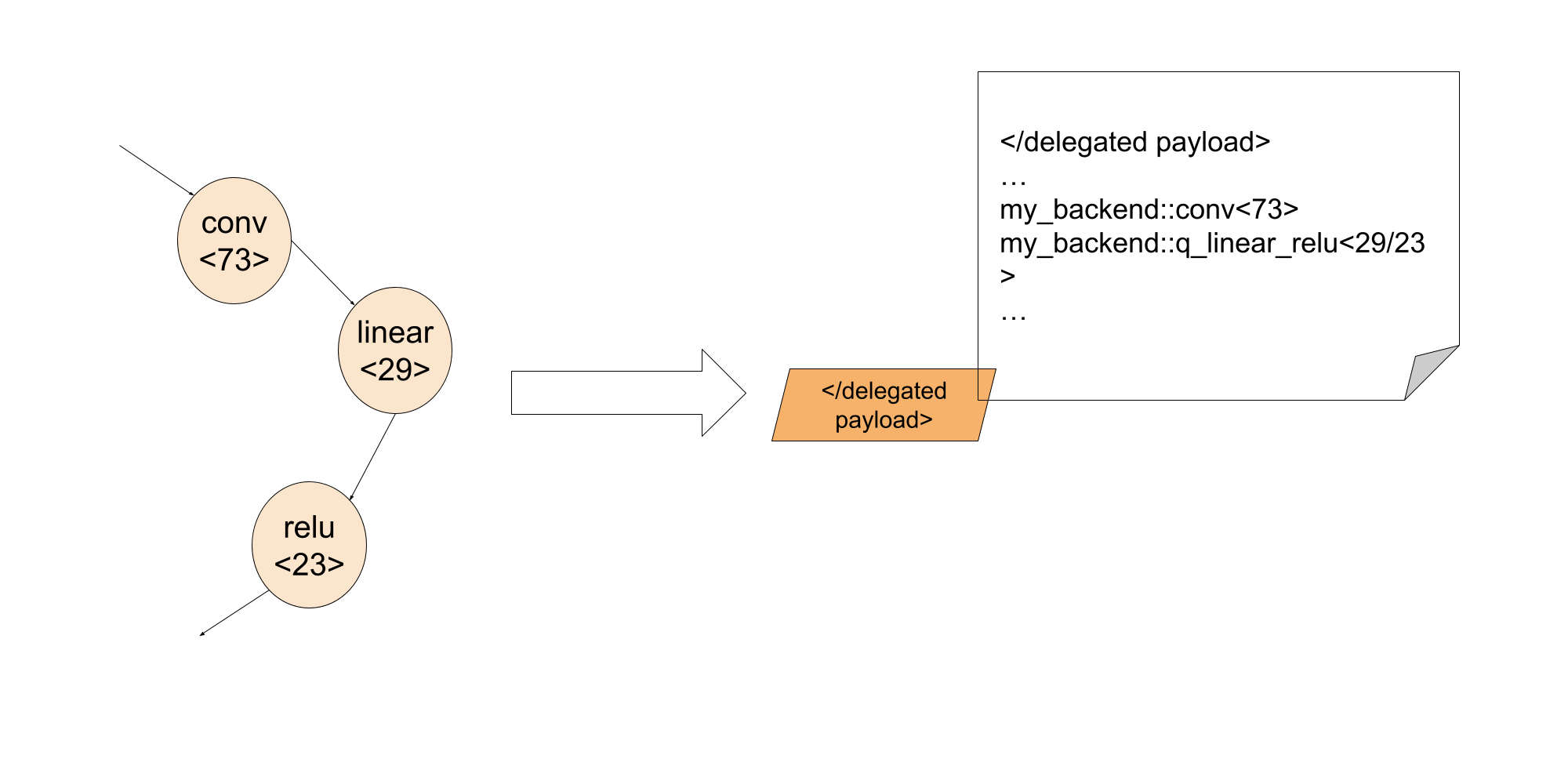
通过这种方式,在执行阶段,使用调试标识符,后端开发者可以将委托内部的失败指令关联到 Python 代码的精确行。
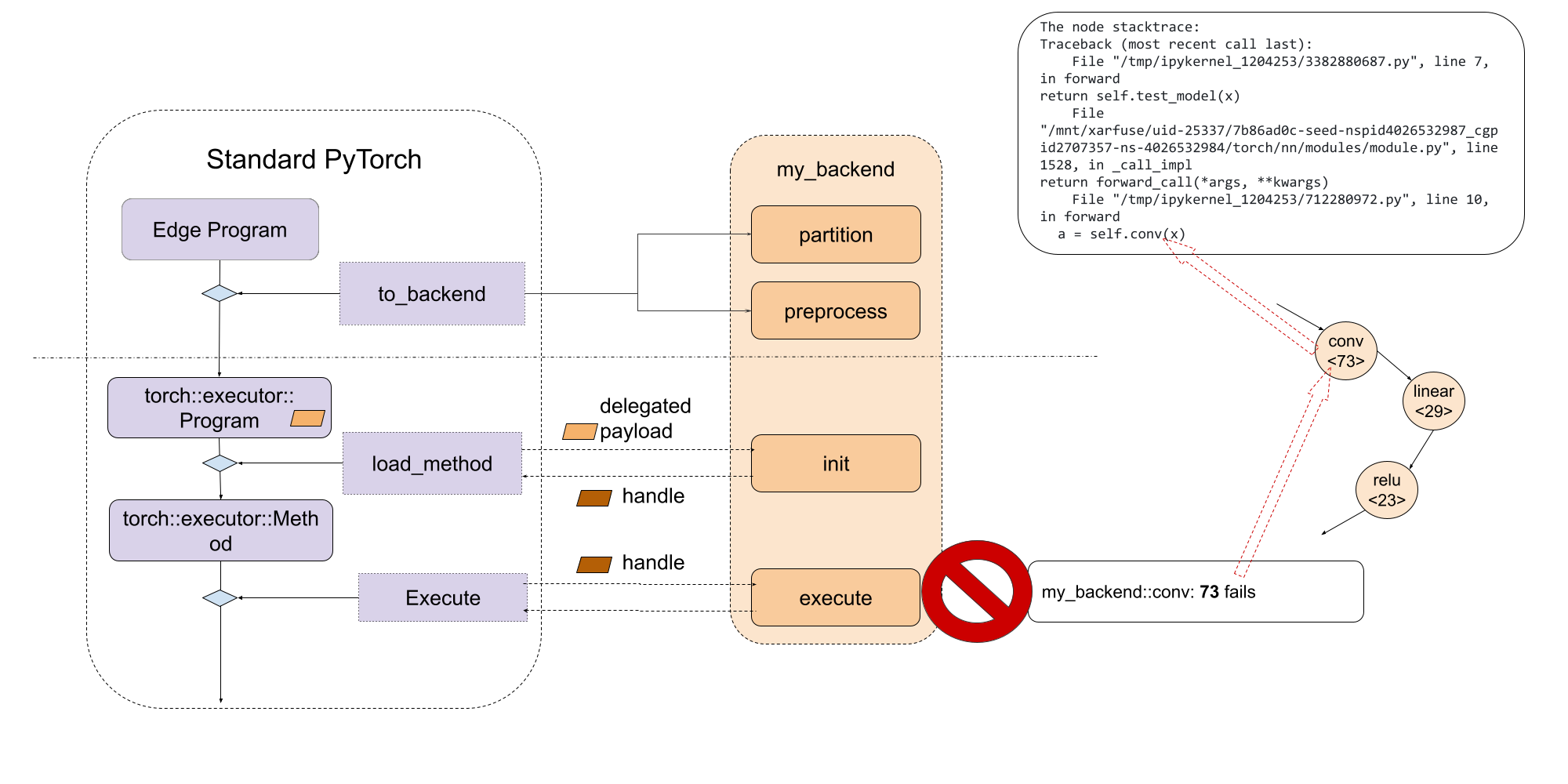
常见问题 ¶
1. 如何在 backend.preprocess 中获取数据?
正在预处理的图模块是一个提升的图,这意味着像权重和偏差这样的静态数据作为输入提供给图。但是,我们可以通过导出程序提前访问权重和偏差。要从给定节点访问这些参数,我们可以使用 torch/_export/utils.py
中提供的 get_params
函数。
2. 如何将数据(如权重/偏差)嵌入到后端?
后端通常有一些方法可以优化常量数据。在这种情况下,我们需要标记作为划分器状态的占位符节点,在 backend.preprocess 期间,我们可以遵循第一个问题的描述来获取权重。
3. 如何使用特定后端在 Python 中运行降低的模块?
我们还没有添加支持,但这是计划!
4. 我们应该期望在边缘方言程序中看到 get_attr
节点吗?
get_attr
节点只会出现在用于控制流或委托的子模块中。它不会保存任何数据。
5. 我们能委托给多个后端吗?
是的!有两种方法可以做到这一点。
选项 1:对不同的后端运行 to_backend 多次
如果我们有两个后端,backend_1 和 backend_2,并且它们有自己的划分器:backend_1_parititioner 和 backend_2_partitioner,我们可以像这样运行它。
# Will first lower nodes to backend_1 depending on the backend_1_parititioner depending on partitioner algorithm
exported_program_backend_1 = to_backend(exported_program, backend_1_parititioner())
# For the rest of nodes, they will be lowered to backend_2 depending on backend_2_parititioner
exported_program_backend_1_and_2 = to_backend(exported_program_backend_1, backend_2_parititioner())
一个更具体的例子可以在 这里 找到。在这个例子中,qnnpack 是一个后端,xnnpack 是另一个后端。我们还没有开源这两个后端委托,这个例子无法开箱即用。它可以作为参考来了解如何实现它。
这个选项很容易尝试,因为通常所有后端都会实现自己的划分器。但是,如果我们更改 to_backend 调用的顺序,这个选项可能会得到不同的结果。如果我们想要更好地控制节点,比如它们应该去哪个后端,选项 2 更好。
选项 2:拥有一个为不同后端进行划分的划分器
另一个选择是创建一个定制的划分器,比如划分器 backend_1_2_partitioner
,并在划分器逻辑内部,
class Backend_1_2_Partitioner(Partitioner):
"""
Partitions all add/mul nodes regardless of order for Backend2
"""
def __init__(self) -> None:
self.delegation_spec_1 = DelegationSpec("Backend1", [])
self.delegation_spec_2 = DelegationSpec("Backend2", [])
self.partition_tags = {}
def partition(
self, exported_program: ExportedProgram
) -> ExportedProgram:
# Tag all nodes in the first partiton to backend 1
node_to_backend_1 = ... # some logic to select the nodes from the graph
delegation_tag = f"backend2_tag{partitioner_1.id}"
node.meta["delegation_tag"] = delegation_tag
self.partition_tags[delegation_tag] = self.delegation_spec_1
# Tag all nodes in the first partiton to backend 2
node_to_backend_2 = ... # some logic to select the nodes from the graph
delegation_tag = f"backend2_tag{partitioner_2.id}"
node.meta["delegation_tag"] = delegation_tag
self.partition_tags[delegation_tag] = self.delegation_spec_2
return exported_program
6. 有没有一种简单的方法来编写一个划分器?
我们提供了一些帮助程序划分器 这里,以方便从分解的操作符中找到节点。
7. 如何将节点链接回源代码? 我们提供了一个帮助程序函数
from executorch.exir.print_program import inspect_node
print(inspect_node(graph, node))
它将突出显示图中的节点并指向源代码,例如输出将如下所示
_param_constant1 error_msg: Here is the node in the graph module:
graph():
%arg0_1 : [num_users=1] = placeholder[target=arg0_1]
%_param_constant0 : [num_users=1] = get_attr[target=_param_constant0]
--> %_param_constant1 : [num_users=1] = get_attr[target=_param_constant1]
%aten_convolution_default : [num_users=2] = call_function[target=executorch.exir.dialects.edge._ops.aten.convolution.default](args = (%arg0_1, %_param_constant0, %_param_constant1, [1, 1], [0, 0], [1, 1], False, [0, 0], 1), kwargs = {})
%_param_constant2 : [num_users=1] = get_attr[target=_param_constant2]
%_param_constant3 : [num_users=1] = get_attr[target=_param_constant3]
%aten_convolution_default_1 : [num_users=1] = call_function[target=executorch.exir.dialects.edge._ops.aten.convolution.default](args = (%aten_convolution_default, %_param_constant2, %_param_constant3, [1, 1], [0, 0], [1, 1], False, [0, 0], 1), kwargs = {})
%aten_add_tensor : [num_users=1] = call_function[target=executorch.exir.dialects.edge._ops.aten.add.Tensor](args = (%aten_convolution_default, %aten_convolution_default_1), kwargs = {})
%_param_constant4 : [num_users=1] = get_attr[target=_param_constant4]
%_param_constant5 : [num_users=1] = get_attr[target=_param_constant5]
%aten_convolution_default_2 : [num_users=1] = call_function[target=executorch.exir.dialects.edge._ops.aten.convolution.default](args = (%aten_add_tensor, %_param_constant4, %_param_constant5, [1, 1], [0, 0], [1, 1], False, [0, 0], 1), kwargs = {})
%aten_gelu_default : [num_users=1] = call_function[target=executorch.exir.dialects.edge._ops.aten.gelu.default](args = (%aten_convolution_default_2,), kwargs = {})
return [aten_gelu_default]
This node _param_constant1 has metadata of:
The node stacktrace:
Traceback (most recent call last):
File "/tmp/ipykernel_1204253/3382880687.py", line 7, in forward
return self.test_model(x)
File "/mnt/xarfuse/uid-25337/7b86ad0c-seed-nspid4026532987_cgpid2707357-ns-4026532984/torch/nn/modules/module.py", line 1528, in _call_impl
return forward_call(*args, **kwargs)
File "/tmp/ipykernel_1204253/712280972.py", line 10, in forward
a = self.conv1(x)