Kubeflow Pipelines¶
TorchX 提供了一个适配器,可以将 TorchX 组件作为 Kubeflow Pipelines 的一部分运行。请参阅 KubeFlow Pipelines 示例.
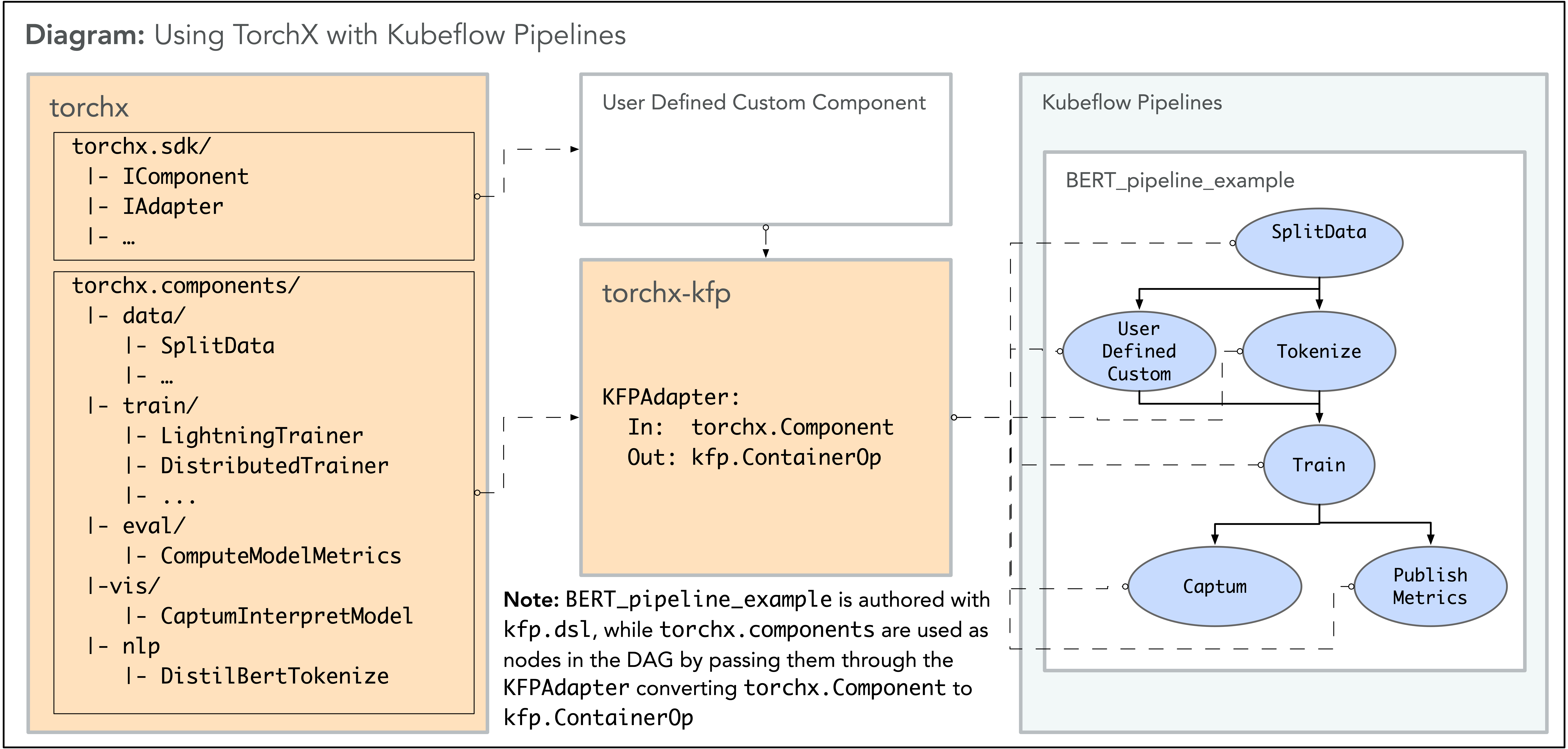
torchx.pipelines.kfp¶

此模块包含用于将 TorchX 组件转换为 KubeFlow Pipeline 组件的适配器。
当前的 KFP 适配器仅支持单节点(1 个角色和 1 个副本)组件。
- torchx.pipelines.kfp.adapter.container_from_app(app: AppDef, *args: object, ui_metadata: Optional[Mapping[str, object]] = None, **kwargs: object) ContainerOp [source]¶
container_from_app 将应用程序转换为 KFP 组件,并返回相应的 ContainerOp 实例。
有关参数的说明,请参阅 component_from_app。任何未指定的参数都会传递给 KFP 容器工厂方法。
>>> import kfp >>> from torchx import specs >>> from torchx.pipelines.kfp.adapter import container_from_app >>> app_def = specs.AppDef( ... name="trainer", ... roles=[specs.Role("trainer", image="foo:latest")], ... ) >>> def pipeline(): ... trainer = container_from_app(app_def) ... print(trainer) >>> kfp.compiler.Compiler().compile( ... pipeline_func=pipeline, ... package_path="/tmp/pipeline.yaml", ... ) {'ContainerOp': {... 'name': 'trainer-trainer', ...}}
- torchx.pipelines.kfp.adapter.resource_from_app(app: AppDef, queue: str, service_account: Optional[str] = None) ResourceOp [source]¶
resource_from_app 从提供的应用程序生成 KFP ResourceOp,该应用程序在 Kubernetes 上使用 Volcano 作业调度程序运行分布式应用程序。有关 Volcano 和如何安装的更多信息,请参阅 https://volcano.sh/en/docs/。
- 参数:
app – 要调整的 torchx AppDef。
queue – 在其中调度操作符的 Volcano 队列。
>>> import kfp >>> from torchx import specs >>> from torchx.pipelines.kfp.adapter import resource_from_app >>> app_def = specs.AppDef( ... name="trainer", ... roles=[specs.Role("trainer", image="foo:latest", num_replicas=3)], ... ) >>> def pipeline(): ... trainer = resource_from_app(app_def, queue="test") ... print(trainer) >>> kfp.compiler.Compiler().compile( ... pipeline_func=pipeline, ... package_path="/tmp/pipeline.yaml", ... ) {'ResourceOp': {... 'name': 'trainer-0', ... 'name': 'trainer-1', ... 'name': 'trainer-2', ...}}
- torchx.pipelines.kfp.adapter.component_from_app(app: AppDef, ui_metadata: Optional[Mapping[str, object]] = None) ContainerFactory [source]¶
component_from_app 接收 TorchX 组件/AppDef 并返回 KFP ContainerOp 工厂。这等效于 kfp.components.load_component_from_* 方法。
- 参数:
app – 要为其生成 KFP 容器工厂的 AppDef。
ui_metadata – 要输出的 KFP UI 元数据,以便您可以在 UI 中显示模型结果。有关格式的更多信息,请参阅 https://www.kubeflow.org/docs/components/pipelines/legacy-v1/sdk/output-viewer/。
>>> from torchx import specs >>> from torchx.pipelines.kfp.adapter import component_from_app >>> app_def = specs.AppDef( ... name="trainer", ... roles=[specs.Role("trainer", image="foo:latest")], ... ) >>> component_from_app(app_def) <function component_from_app...>
- torchx.pipelines.kfp.adapter.component_spec_from_app(app: AppDef) Tuple[str, Role] [source]¶
component_spec_from_app 从 TorchX 组件中获取并生成其 yaml 规范。值得注意的是,它不会应用资源或端口映射,因为这些必须在运行时应用,这就是它也返回角色规范的原因。
>>> from torchx import specs >>> from torchx.pipelines.kfp.adapter import component_spec_from_app >>> app_def = specs.AppDef( ... name="trainer", ... roles=[specs.Role("trainer", image="foo:latest")], ... ) >>> component_spec_from_app(app_def) ('description: ...', Role(...))